The complete junior to senior developer - Notes
Ssh (secure shell) - Protocol
It's a protocol to use over shell, the significant advantage offer by SSH over its predecessors is the use of encryption to ensure secure transfer of information between host and the client.
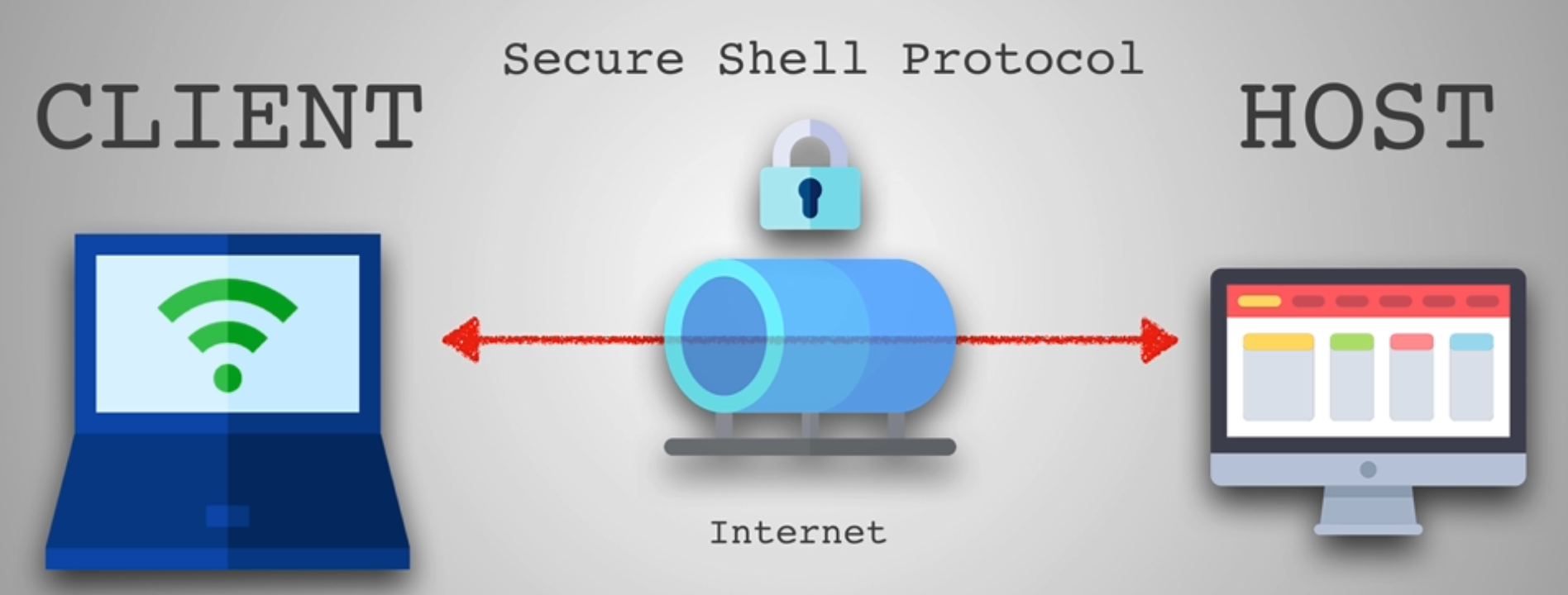
The host refers to the remote server you're trying to access, while the client is the computer you're using to access the host.
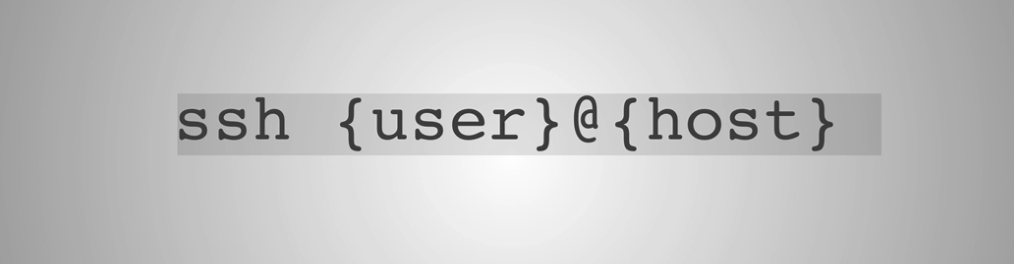
Symmetrical Encryption
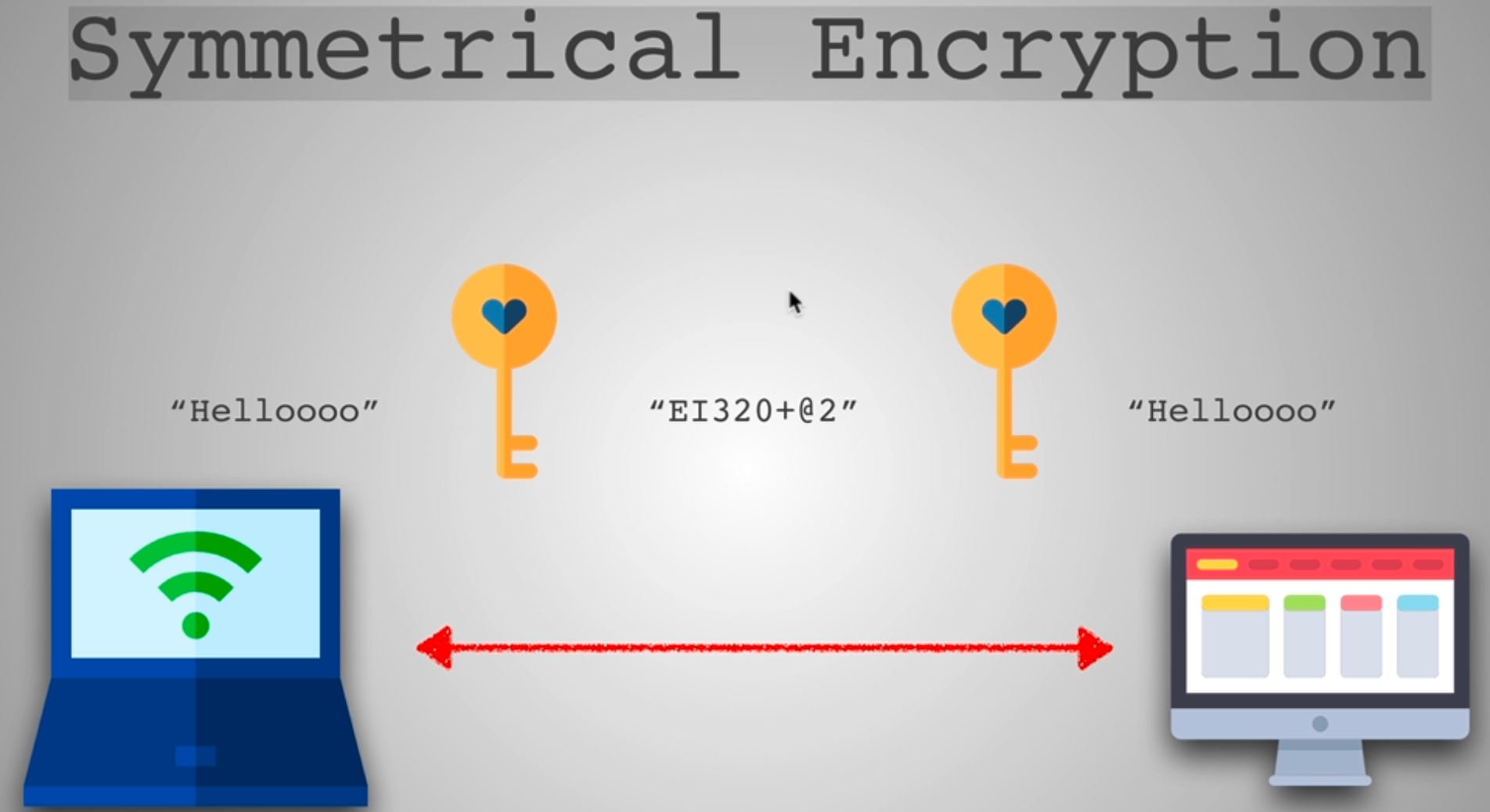
key exchange algorithm - A secure way to exchange these keys without any bad actors intercepting it. the key is never actually transmitted between the client and the host. Instead, the two computers share public pieces of data and then manipulate it to independently calculate the secret key.
Asymmetrical Encryption
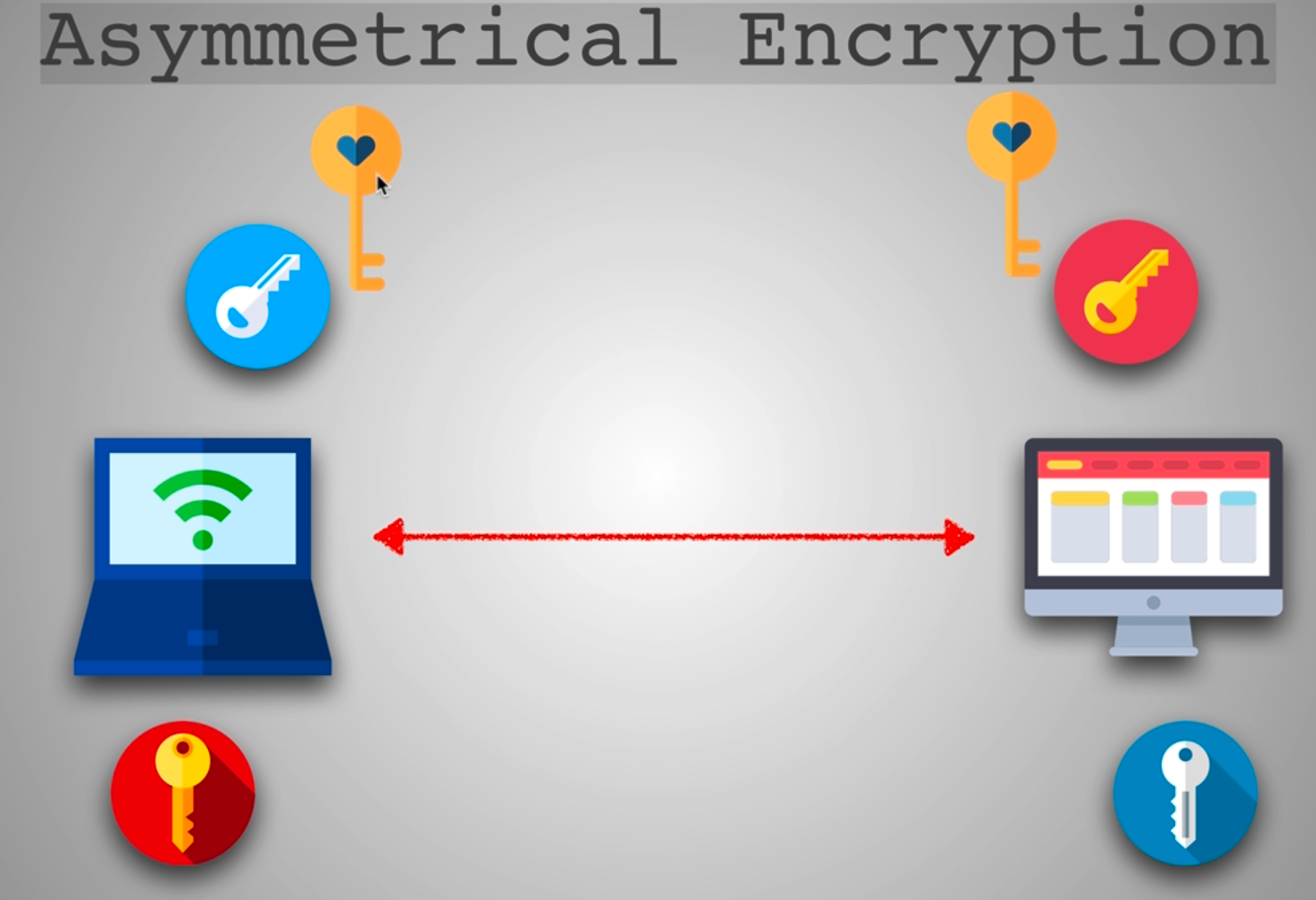
Diffie Hellman Key Exchange - what it does basically is that it uses a bit of this information a bit of the public keys to generate without ever exchanging the keys. Each machine on its computer can generate this symmetric key from data from each computer. makes it possible for each party to combine their own private data with public data from other systems to arrive at an identical secret session key.
Hashing
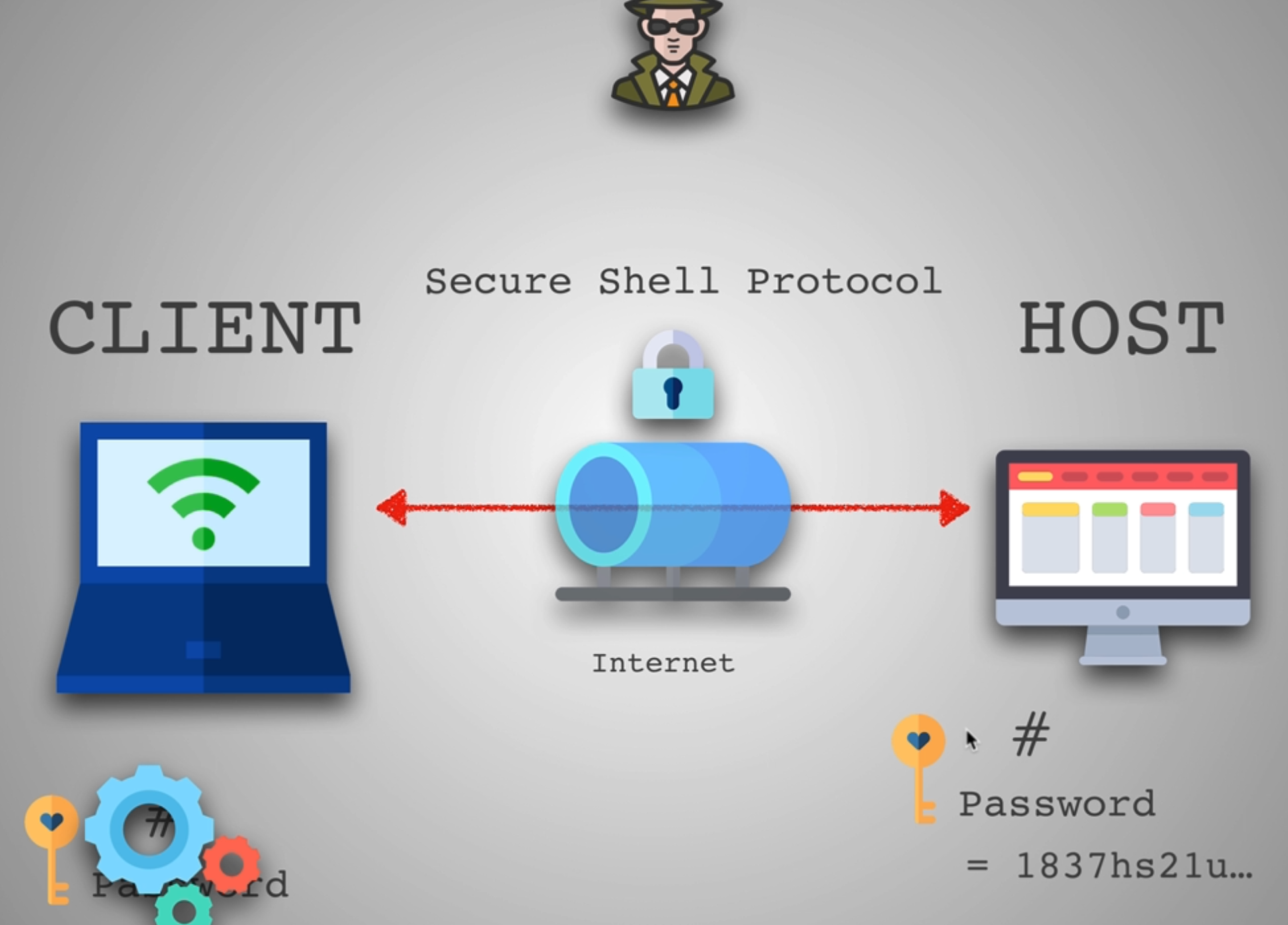
HMX (hash based message authentication codes)
So how can the host check that the message hasn't been tampered with. Well what they can do now is because they have the same information they can use their own symmetric key which is the same as the clients. They can use the packet sequence number again which they both know and then because this message was sent through Ssh they also have that message that was sent. So now they run it through the same hash function again. And once it calculates it it's going to see does what I just generated match what the client hash was. And if it does match well that means that this message was not tampered
Optimize
Jpeg - used for photos, images and things with many colors such as photographs. Downside is that they don’t allow for transparency.
Gif - limit total colors that you can use in a GIF somewhere between 2 to 256 and reducing the color count least to huge false savings. Good for small animation.
Png - limits the number of colors you can use and they tend to be a lot smaller in size than JPG. can add transparancy.
Svg - is a completely different category from all the other 3 file format, there are actually something call vector graphics. It can be expand to several times its original size and it will be just as sharp as the original. Incredible small good for 4k displays.
https://99designs.com/blog/tips/image-file-types/
https://www.sitepoint.com/gif-png-jpg-which-one-to-use/
Http/2
In theory it can have one connection for something called parallelism so 1 connection has many files sent into it.
Http/3
React + Redux + Module Bundling
State is simply an object that describe of your app.
npm install -g create-react-app
create-react-app robofriends
export const robots = [
{
id: 1,
name: 'Leanne Graham 1',
username: 'Bret 1',
email: '[email protected]'
},{
id: 2,
name: 'Leanne Graham 2',
username: 'Bret 2',
email: '[email protected]'
},{
id: 3,
name: 'Leanne Graham 3',
username: 'Bret 3',
email: '[email protected]'
},{
id: 4,
name: 'Leanne Graham 4',
username: 'Bret 4',
email: '[email protected]'
}
]
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './containers/App'
import 'tachyons';
//import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
//import { robots } from './robots'
import CardList from '../components/CardList'
import SearchBox from '../components/SearchBox'
import Scroll from '../components/Scroll'
import { Component } from 'react'
class App extends Component {
constructor() {
super()
this.state = {
robots: [],
searchfield: ''
}
}
componentDidMount() {
fetch('<https://jsonplaceholder.typicode.com/users>')
.then(response => response.json())
.then(users => this.setState({robots: users}));
}
onSearchChange = (event) => {
this.setState({ searchfield: event.target.value})
}
render() {
const { robots, searchfield } = this.state;
const filteredRobots = robots.filter(robot => {
return robot.name.toLowerCase().includes(searchfield.toLowerCase());
})
return !robots.length ?
<h1>Loading</h1> :
(
<div className="tc">
<h1>Robofriends</h1>
<SearchBox searchChange={this.onSearchChange} />
<Scroll>
<CardList robots={filteredRobots} />
</Scroll>
</div>
)
}
}
export default App;
import Card from './Card'
const CardList = ({robots}) => {
return (
<div >
{
robots.map((user,i) => {
return (
<Card key={i} id={robots[i].id} name={robots[i].name} email={robots[i].email}/>
);
})
}
</div>
);
};
export default CardList;
const Card = ({ name, email, id }) => {
return (
<div className="bg-light-green dib br3 pa3 ma2 grow bw2 shadow-5 tc">
<h1>Robofriends</h1>
<img alt="robots" src={`https://robohash.org/${id}?200x200`} />
<div>
<h2>{name}</h2>
<p>{email}</p>
</div>
</div>
)
}
export default Card;
const SearchBox = ({searchfield, searchChange}) => {
return (
<div className="pa2">
<input
className="pa3 ba b--green bg-lightest-blue"
type="search"
placeholder="search robots"
onChange={searchChange}
/>
</div>
);
}
export default SearchBox;
const Scroll = (props) => {
console.log(props);
return (
<div style={{overflowY:'scroll', border: '1px solid black', height: '800px'}}>
{props.children}
</div>
)
}
export default Scroll;
State management
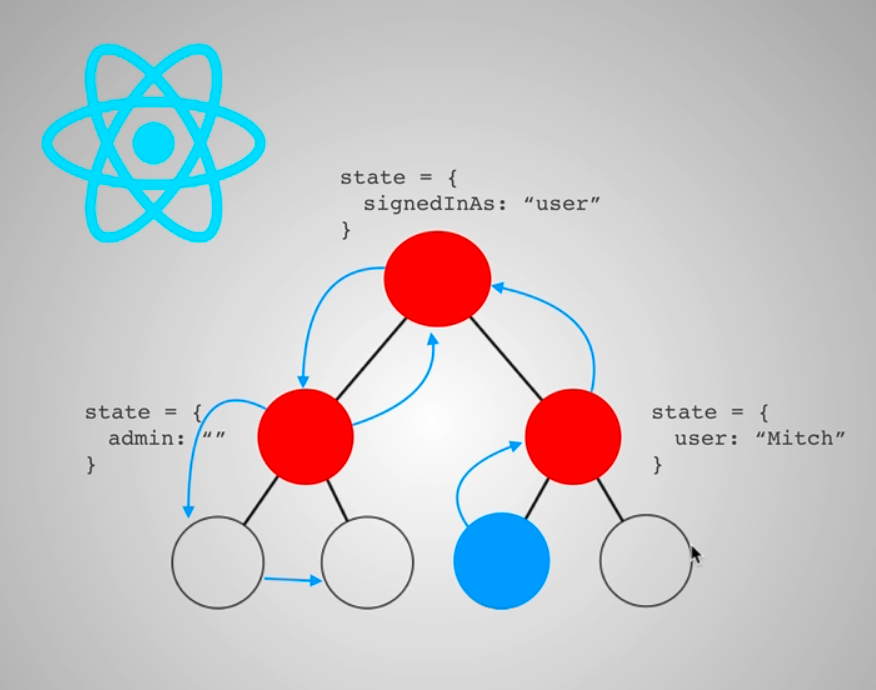
no state management
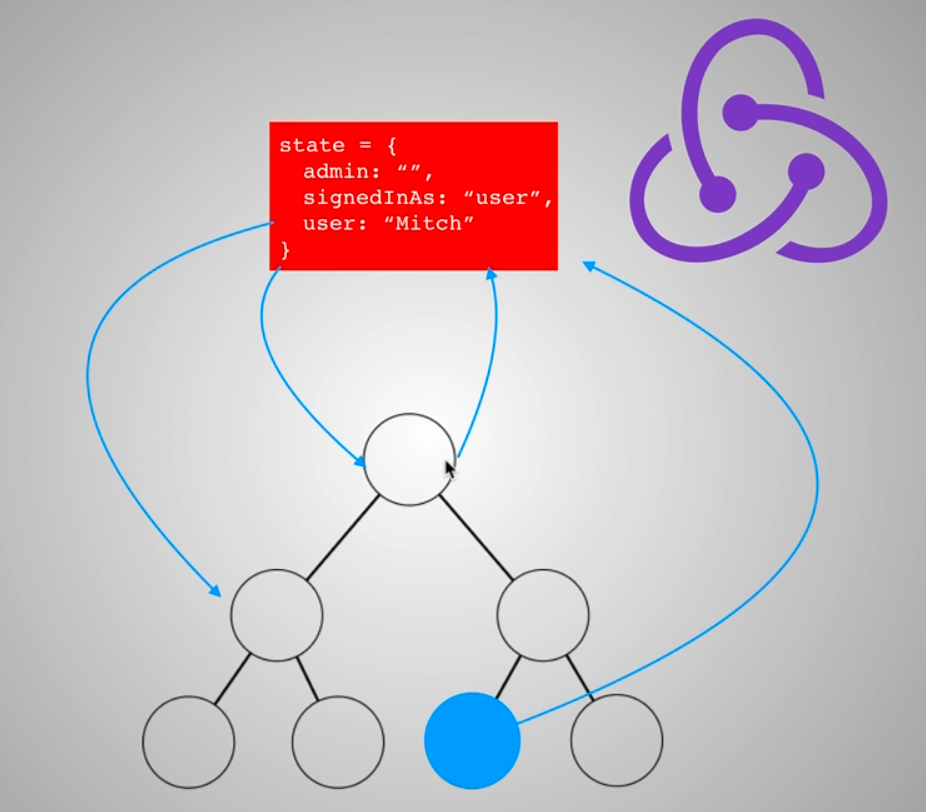
state management using redux
Why redux?
- good for managing large state
- useful for sharing data between containers
- predictable state management using the 3 principles
The 3 principles
- single source of truth
- state is read only
- changes using pure functions
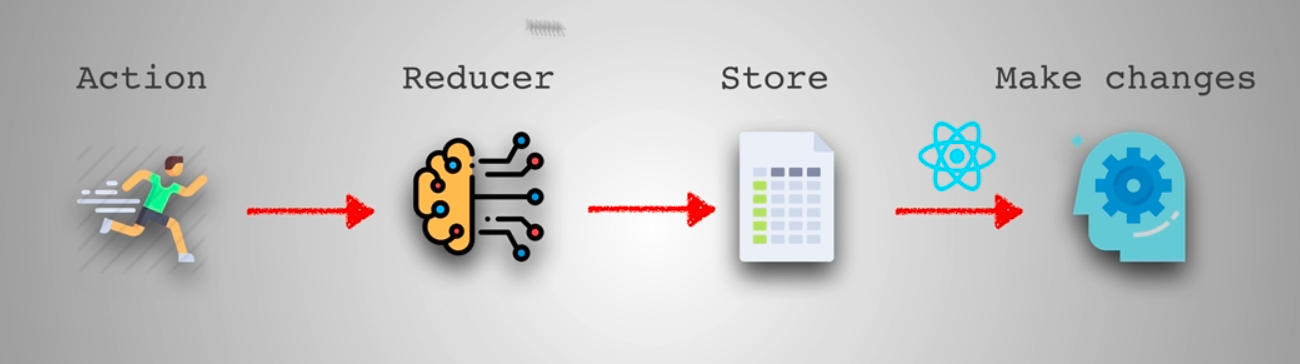
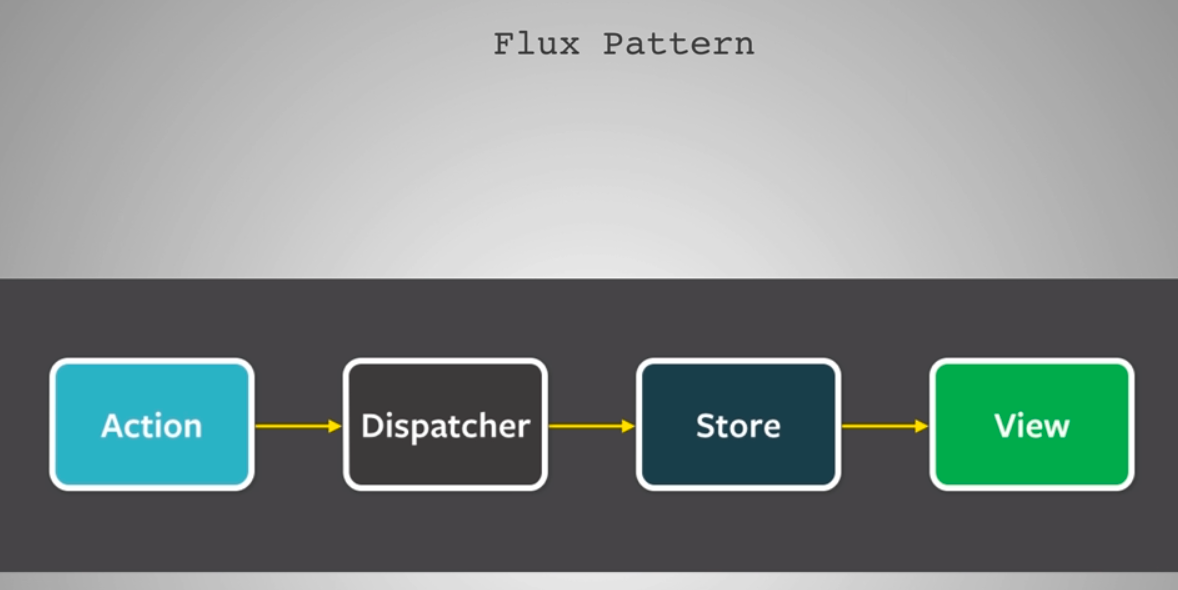
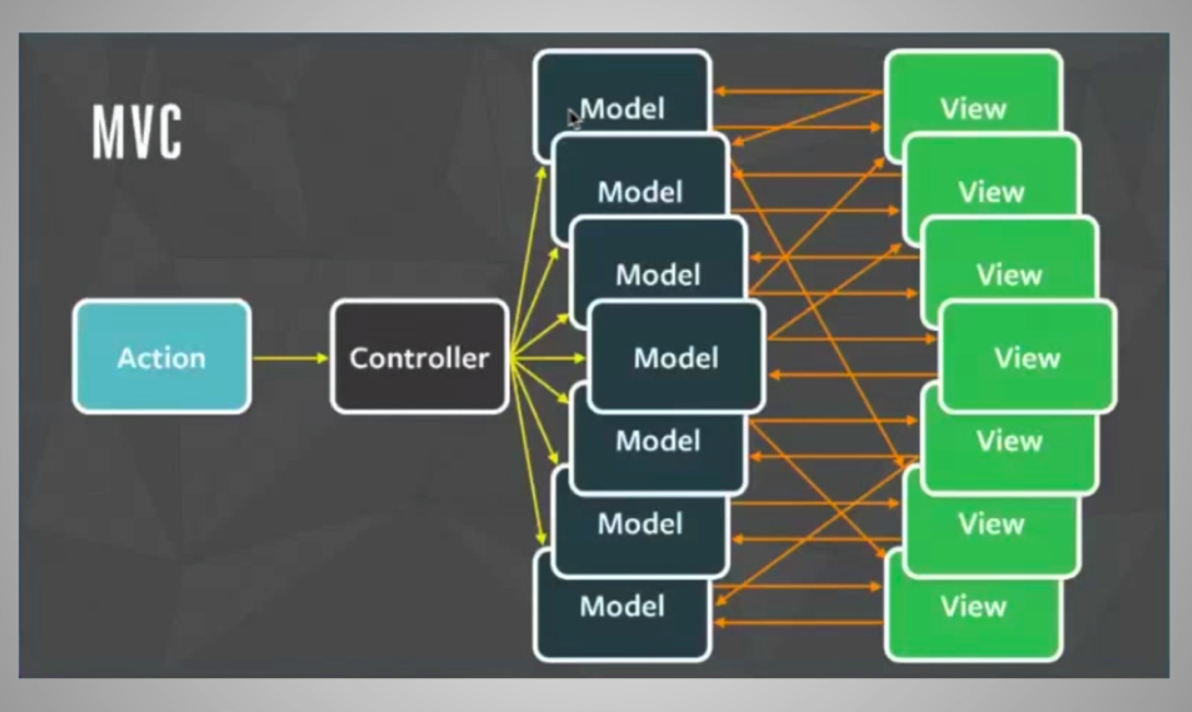
How javascript works
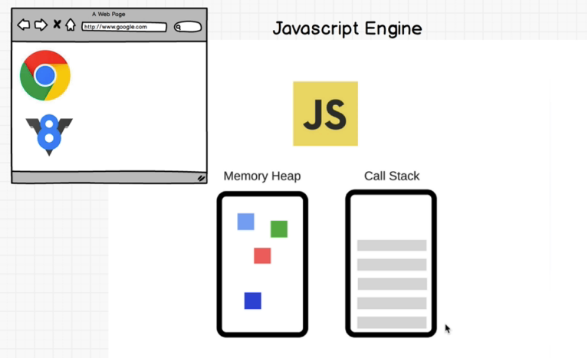
Javascript is a single-threaded language that can be non-blocking, has 1 call stack FIFO
synchronous programming means each line gets executed.
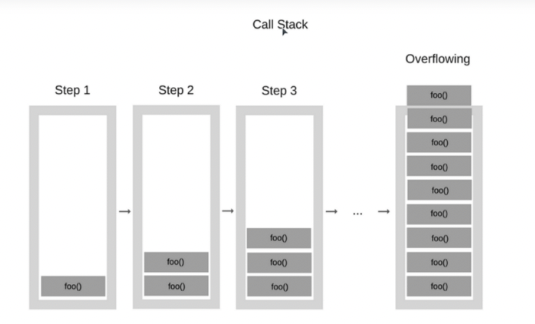
Stackoverflow
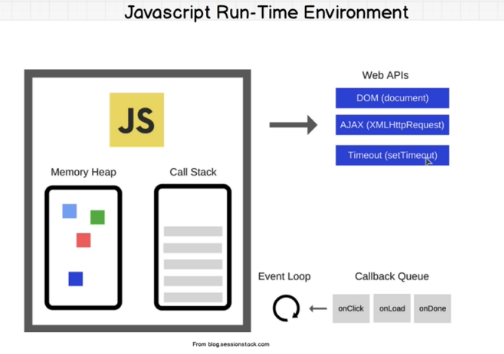
Redux
npm install redux
npm install react-redux
Using provider, connect.
import { Provider, connect } from 'react-redux';
import { createStore } from 'redux';
import { searchRobots } from './reducers';
const store = createStore(searchRobots);
<Provider store={store}>
<App />
</Provider>
Performance
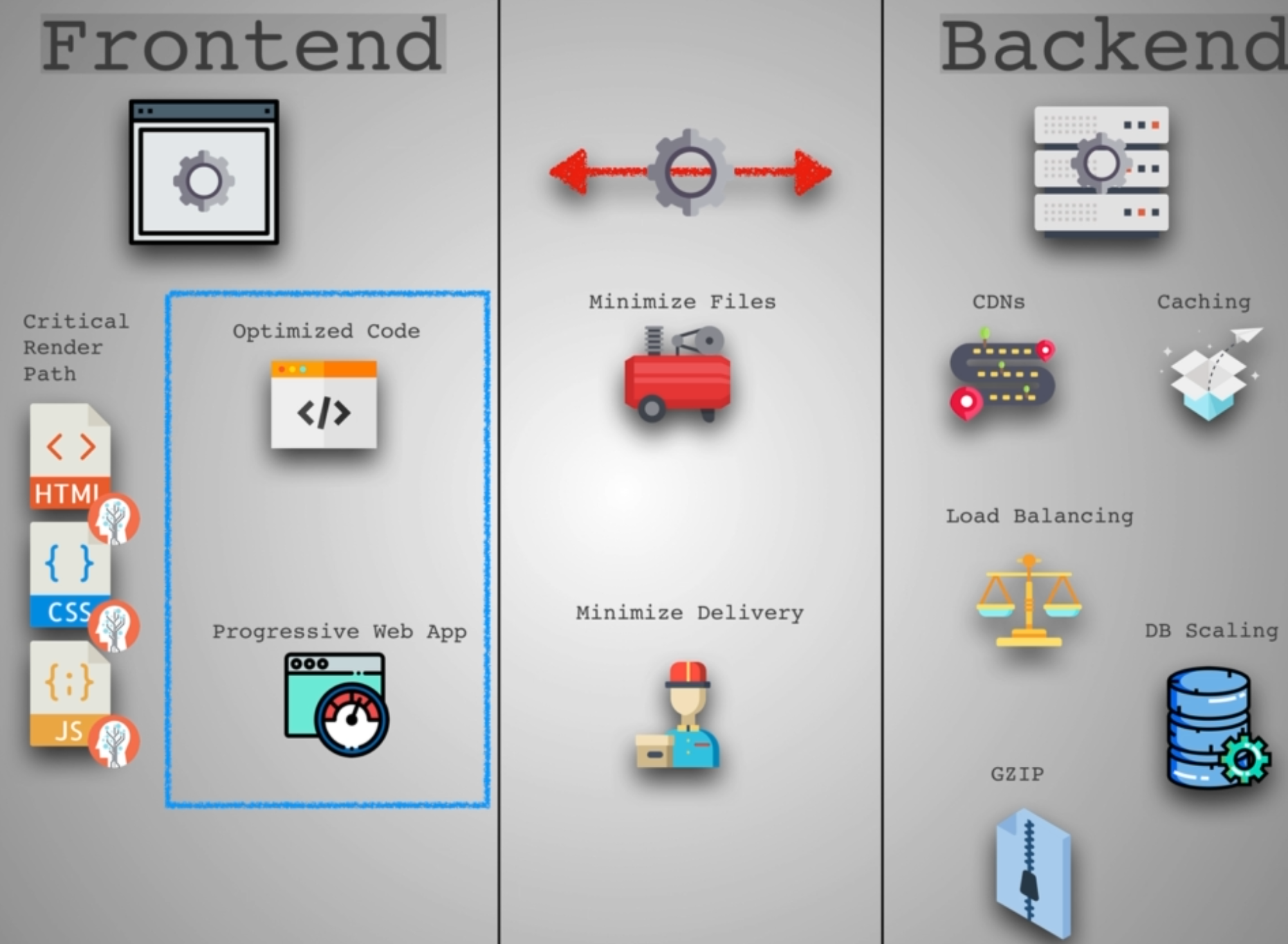
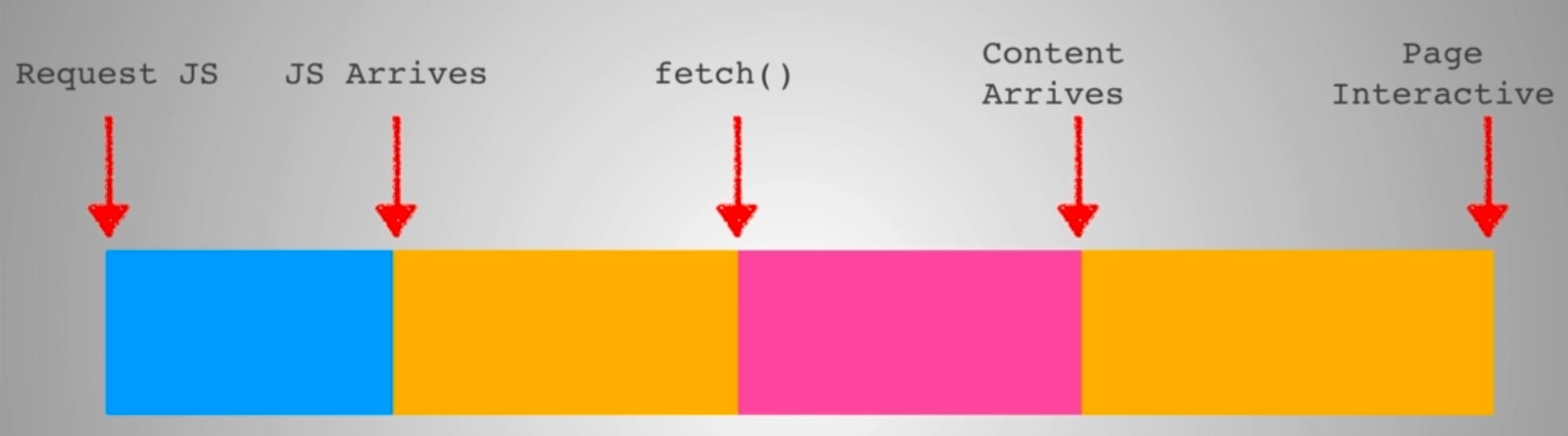
tools - webpagetest.org
Spa vs Server Side
Example : producthunt.com (SPA)
Cons SPA - Load initial takes a lot & SEO
CSR vs SSR
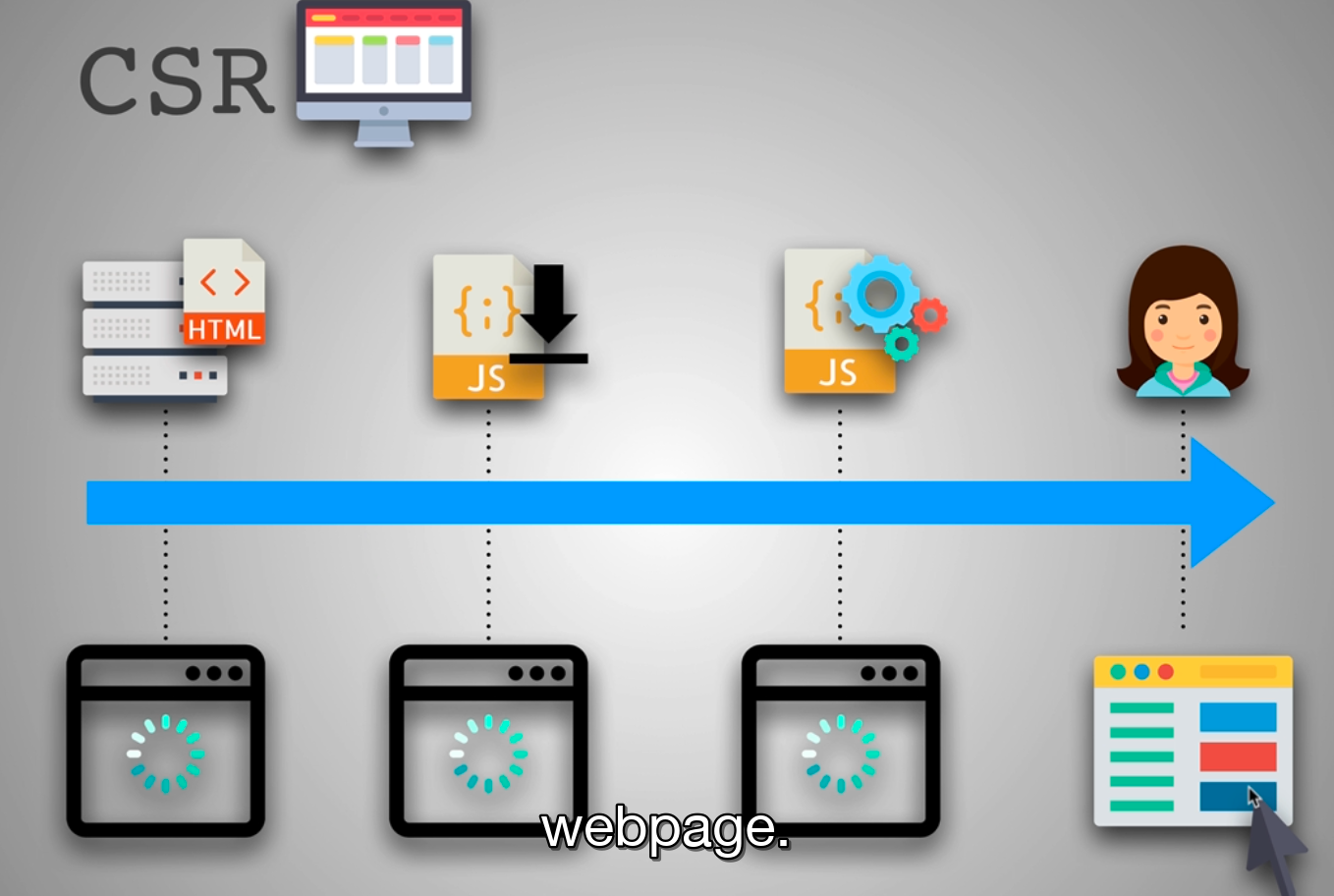
Pros - Rich interaction, faster response, web application
Cons - Low SEO potential, Longer initial load
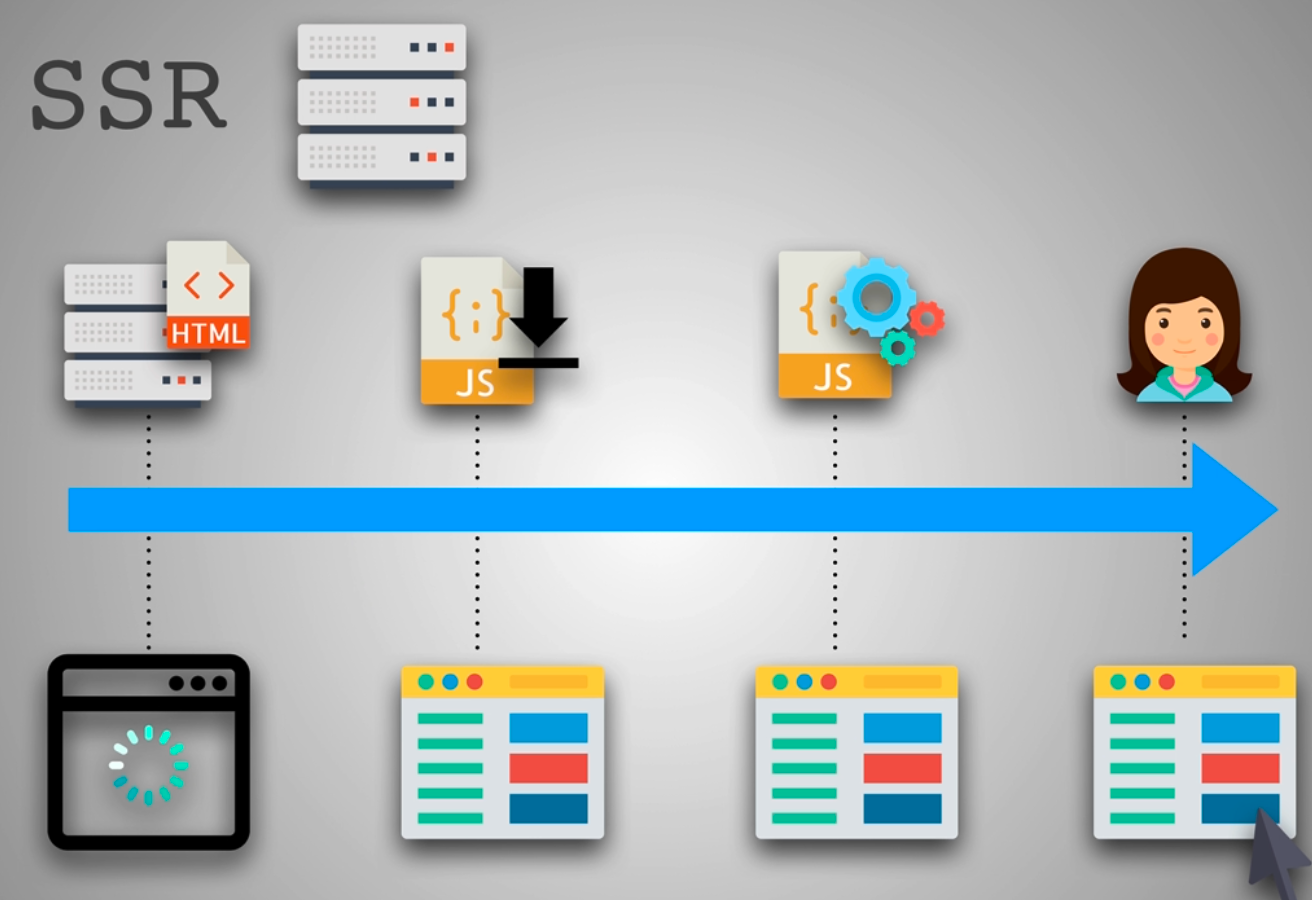
Pros - Static sites, SEO, Initial Page Load
Cons - Full page Reloads, Slower page rendering
SSR Next
npm install next react react-dom
npm install isomorphic-unfetch
import Link from 'next/link';
import fetch from 'isomorphic-unfetch';
const Robots = (props) => {
return (
<div>
<h1>Robots</h1>
<Link href="/">
Index
</Link>
<Link href="/about">
About
</Link>
<Link href="/robots">
Robots
</Link>
<div>
{
props.robots.map(robot => (
<li key={robot.id}>
<Link href={`robots/${robot.id}`}>
{robot.name}
</Link>
</li>
))
}
</div>
</div>
)
}
Robots.getInitialProps = async function() {
const res = await fetch('<https://jsonplaceholder.typicode.com/users>')
const data = await res.json()
return {
robots: data
}
}
export default Robots;
Security
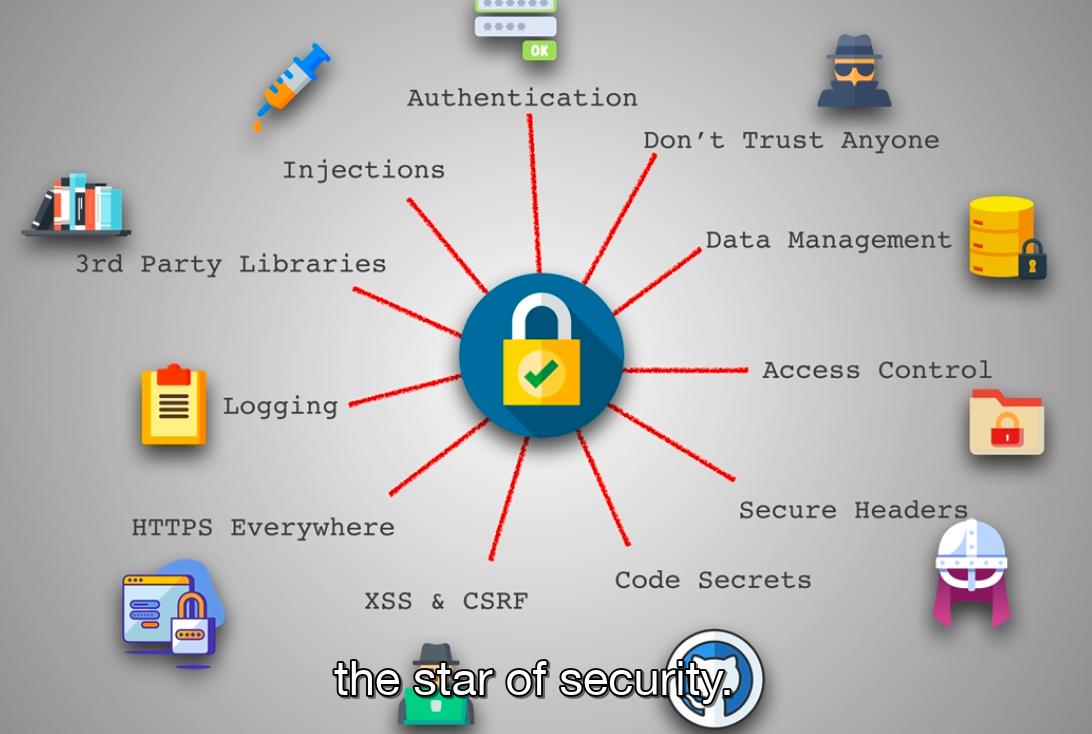
Injections
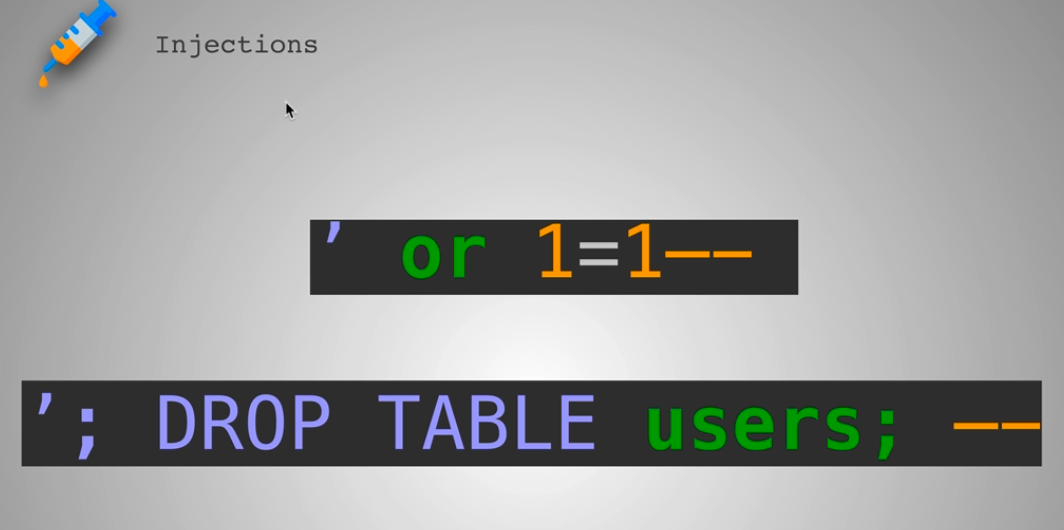
Sanitize input, Parametrize Queries, Knex.js or other ORMS
https://www.hacksplaining.com/exercises/sql-injection
3rd party libraries
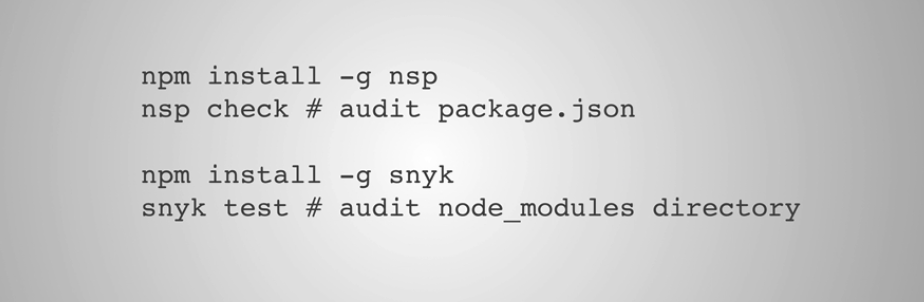
nsp to audit package.json
synk to audit node_modules
Logging
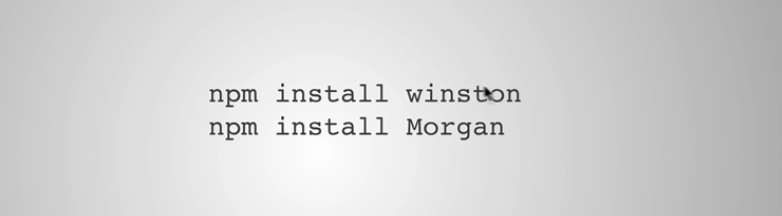
Morgan use as middleware to log http
Winston a logger use for everything ( like console.log) but winston.log(’info - ‘)
Https
lets encrypt & cloudflare
XSS & CSRF
Cross site scripting occurs whenever an application includes untrusted data in a anew page without proper validation or escaping or updating a web page with user supplied data using javascript.
It allows an attacker to execute scripts in a victim’s browser
fetch(’//httpbin.org/post’, {method: ‘POST’, body: document.cookie})
#Example using nodejs
app.get('/', (req,res) => {
res.cookie('session', '1', { httpOnly: true })
res.cookie('session', '1', { secure: true })
res.set({
'Content-Security-Policy": "script-src 'self' '<https://apis.google.com>'",
})
})
# in console to test it out
document.write('<script>alert("test");</script>')
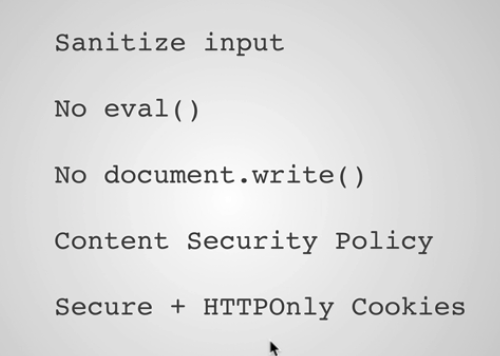
exercise xss - https://www.hacksplaining.com/exercises/xss-stored
protecting your users against cross-site scripting
exercise csrf - https://www.hacksplaining.com/exercises/csrf
protecting your users against csrf
Code secret
Example - Environment Variables, commit history
Secure Headers
https://github.com/helmetjs/helmet
npm install helmet
const helmet = require('helmet');
app.use(helmet());
HTTP: The Protocol Every Web Developer Must Know—Part 1
Access Control
Principal of least privilege
Data Management
bcrypt, scrypt, Aragon2
pgcrypto - encrypt a few columns
Don’t trust anyone
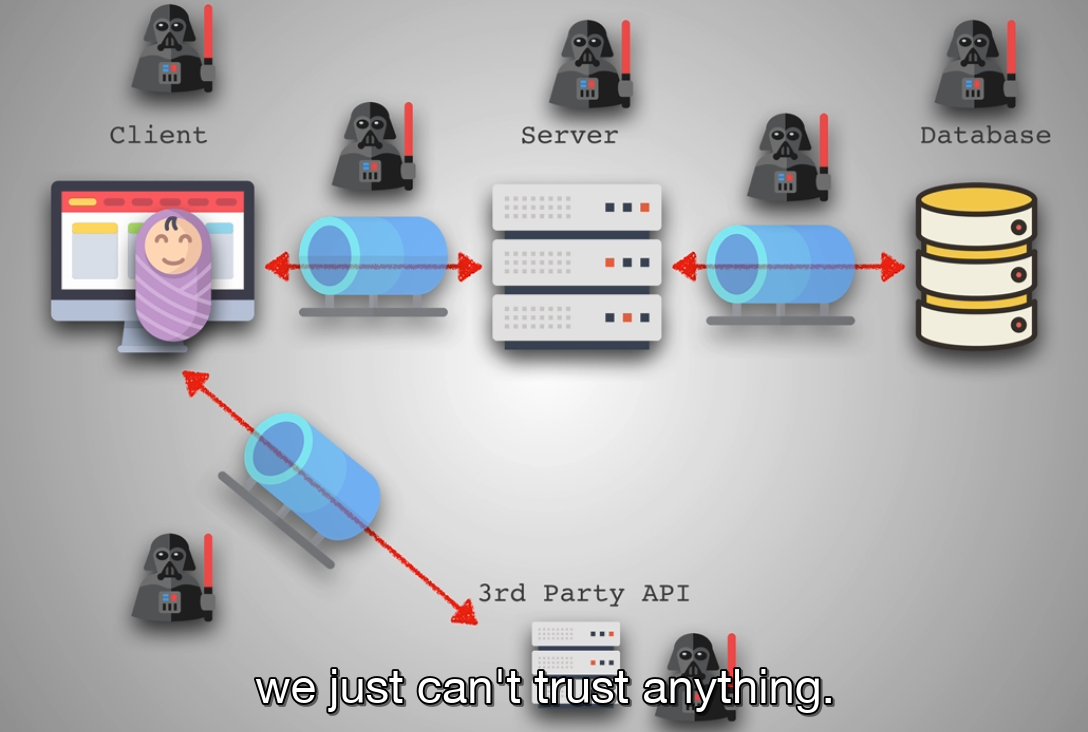
Authentication
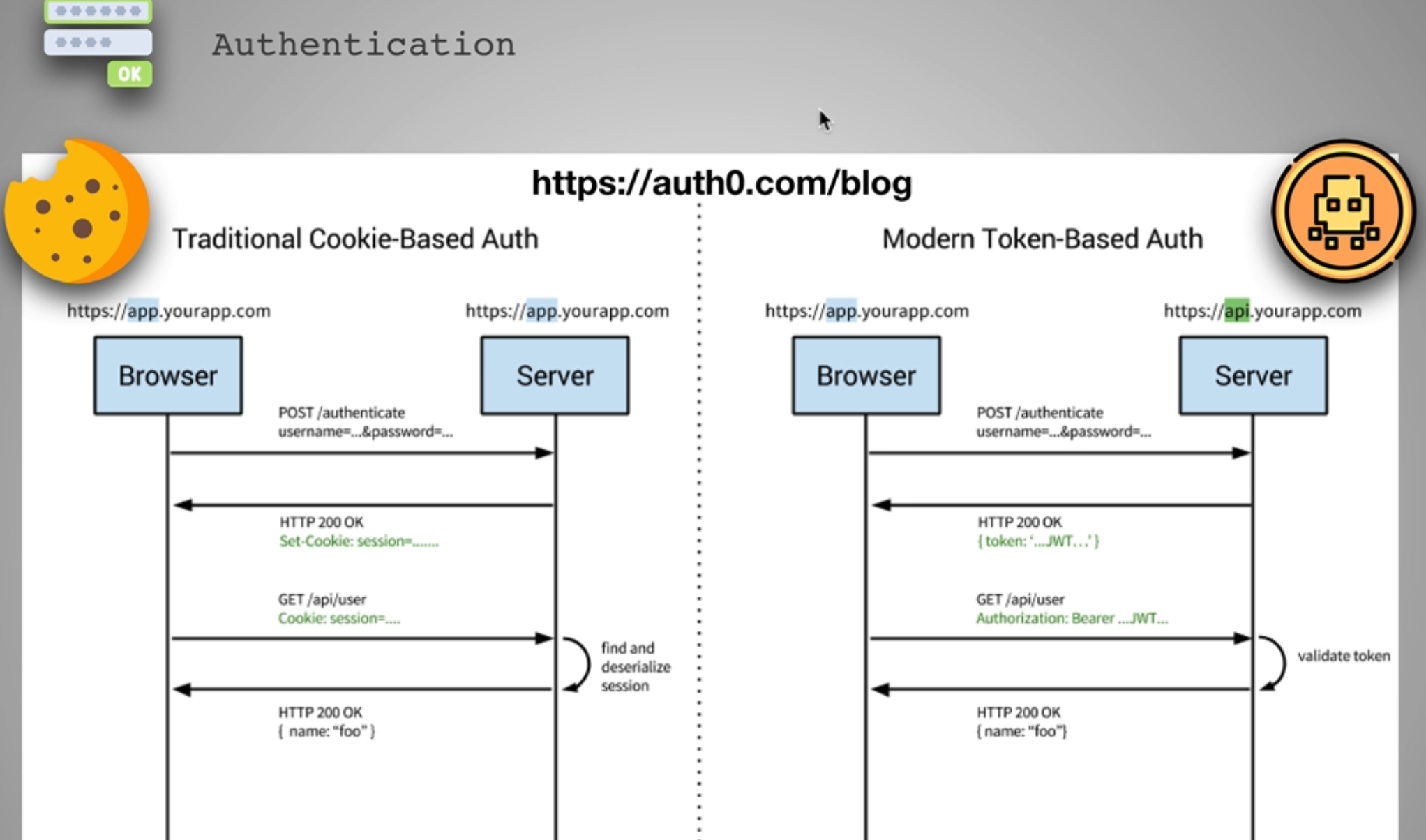
https://www.hacksplaining.com/lessons
AWS
Serverless
sudo npm install -g serverless
serverless create -t aws-nodejs // will create files
serverless configcredentials --provider aws --key {iamkey} --secret {iamsecret} // will create .aws
'use strict';
const emojis = [
'😄','😃','😀','😊','😉','😍','🔶','🔷', '🚀'
];
module.exports.hello = (event, context, callback) => {
const rank = event.queryStringParameters.rank;
const rankEmoji = emojis[rank > emojis.length ? emojis.length - 1 : rank];
const response = {
statusCode: 200,
headers: {
"Access-Control-Allow-Origin" : "*" // Required for CORS support to work
},
body: JSON.stringify({
message: 'Rank generated!',
input: rankEmoji,
}),
};
callback(null, response);
// Use this code if you don't use the http event with the LAMBDA-PROXY integration
// callback(null, { message: 'Go Serverless v1.0! Your function executed successfully!', event });
};
Performance
Database Scaling
- Identify inefficient queries
- Increase memory
- vertical scaling (redis, memcached)
- sharding
- more databases
- database type
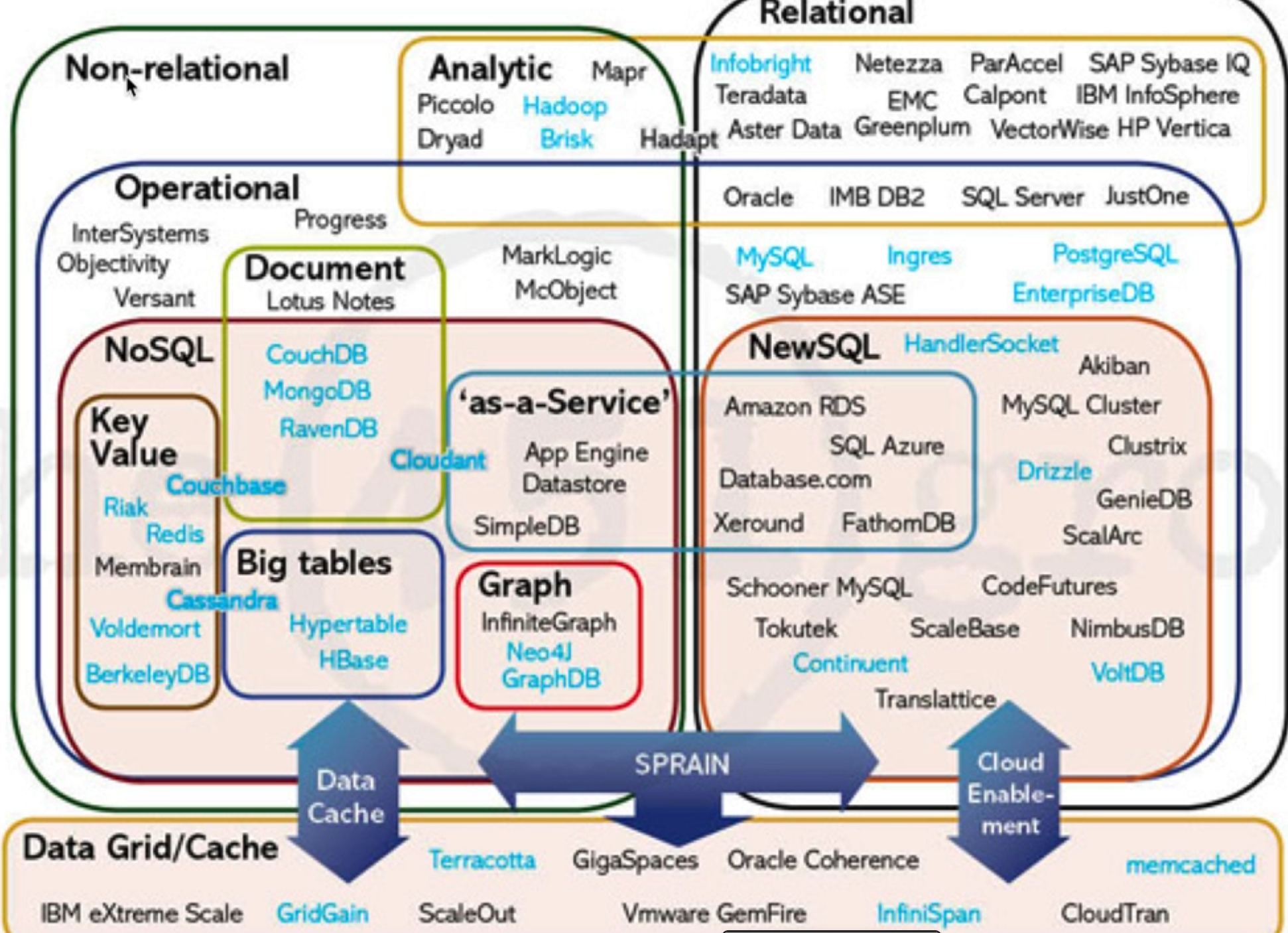
Caching
Caching is the process of storing some data in cache, cache is a temporary storage component are where the data is stored so that in future can be served faster.
Load balancing
it’s a way for us to balance multiple request at the same time and distributed them to different services.
// test concurrent users
npm install -g loadtest
loadtest -t 1 -c 100 --rps 100 <http://localhost:8000>
CI / CD
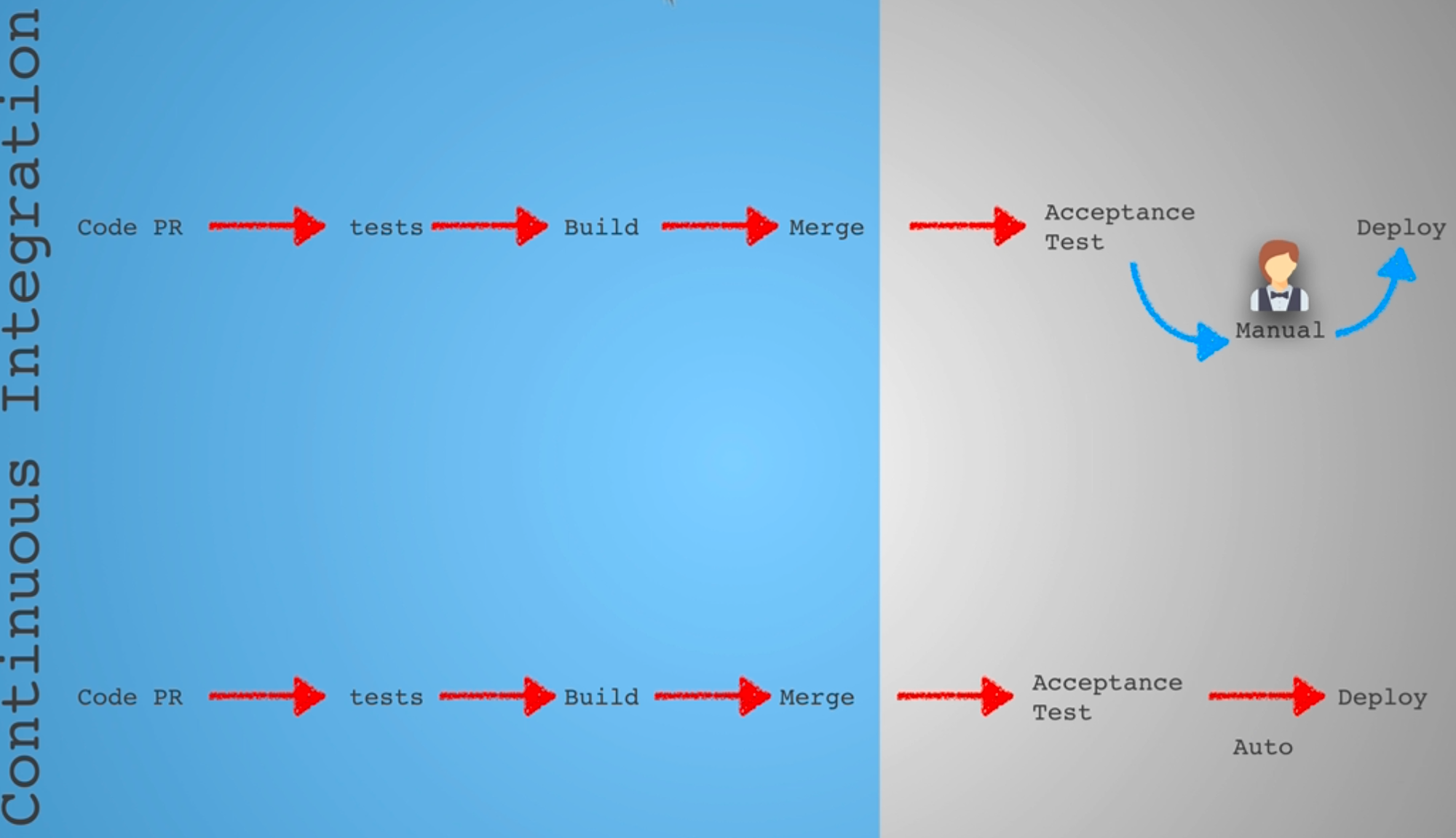
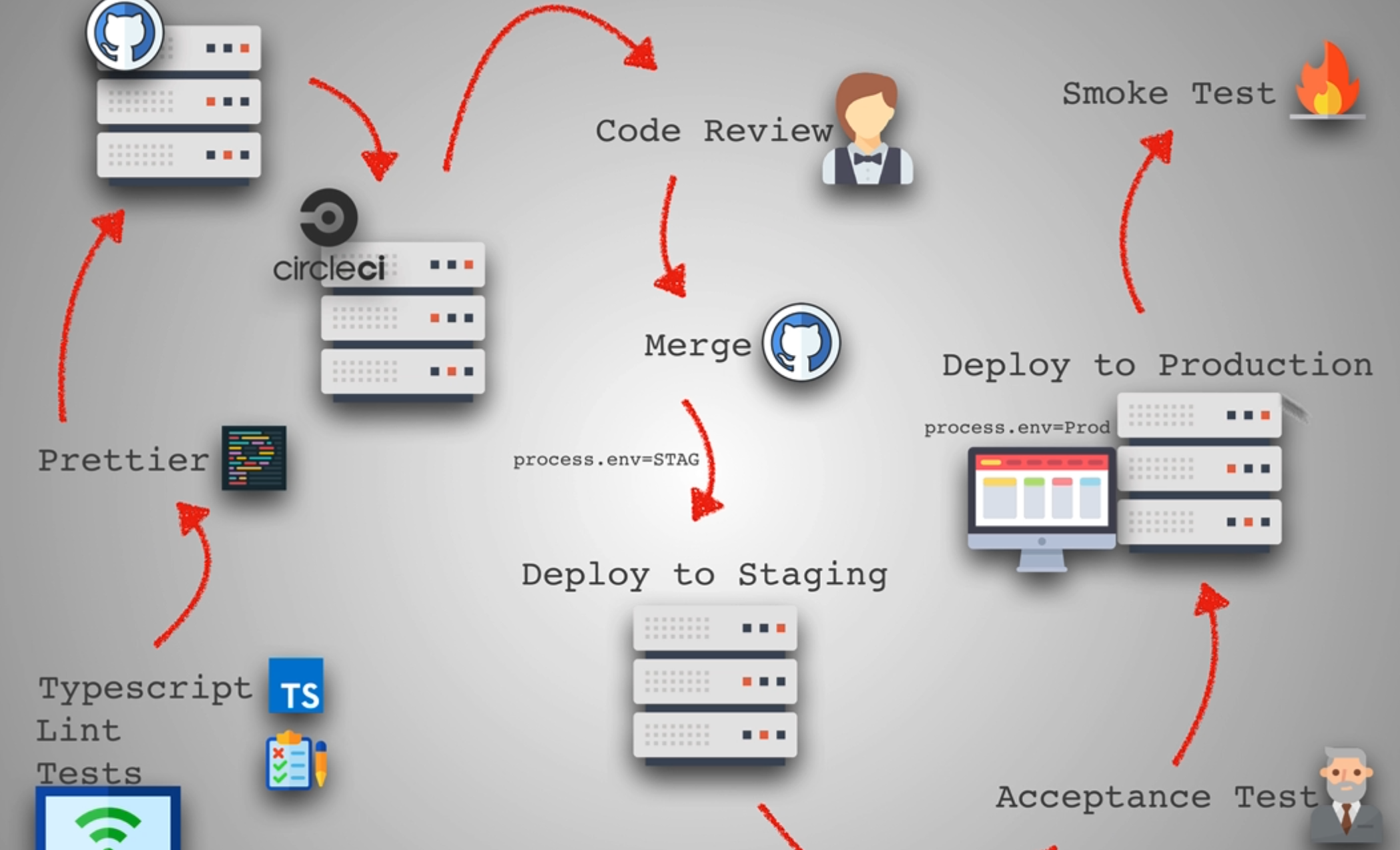