Laracon Summer 2022 - Notes
Laracon Summer notes
Laracon Online Summers
Not Quite My Type - Kai sassnowski
public function wait(Duration $duration): void
{
if ($seconds < 0) {
throw new InvalidArgumentException();
}
}
final class Duration {
public function __construct(
public readonly int $seconds
) {
throw new InvalidArgumentException();
}
public static function seconds(int $seconds): self {
return new self($seconds);
}
}
$clock->wait(Duration::seconds(5));
$clock->wait(-5);
$clock->wait(Duration::seconds(-5));
class ShoppingCart
{
private ?Discount $discount = null;
public function __construct(
private array $products
) {
public function total(): int {}
public function applyDiscount(Discount $discount): void
{
$this->discount = $discount;
}
}
}
final class Discount {
public function __construct(
public readonly float $percentage
) {
if ($percentage <= 0 || $percentage > 1.0) {
throw new InvalidArgumentException();
}
}
public static function percentOff(int $value): self {
return new self($value/100);
}
}
$cart->applyDiscount(Discount::percentOff(25));
My notes : a bit of overengineering, nice to know these concepts of types.
Check out:
phpsandbox.io, play.phpsandbox.io
Need to look up:
Phpstan, Psaml
model casts concept
Kubernetes and Laravel - Bosun Egberinde
is an open source system for automating deployment, scaling and management of containerized applications.
img1
How k8s do what it does
the k8s cluster → cluster, nodes, control planes.
node → kubelet, kubeProxy, Container Runtime
Control plane → scheduler, api server, etcd, cc, controller manager,etc
img2
Creating your own k8s cluster
- Kind - containerized
- MiniKube - VM based
Cloud provided k8s cluster
- GKE on google cloud
- EKS on AWS
Kubernetes Objects
Pods, Deployments, services, ingresses, replicasets, configmaps
Manipulating K8s objects
Kubectl, Kubernetes Dashboard, Kubernetes API
kubectl get pods
kubectl apply -f dep.yaml
kubectl get deployments
kubectl exec -it {pods} -- ash
Sustainable self care - Marje Holmstrom-sabo
Definitions - shared meaning - sustainable, self-care, mind, body, spirit, community.
Sustainable - using things wisely so you don’t use them up when you’re done.
Self care - care for oneself
Mind - the element or complex of elements in an individual that feels, perceives, thinks, wills, and especially reasons.
body - organized physical substance of an animal or plant either living or dead.
spirit - an animating or vital principal held to give life to physical organisms.
community - A unified body of individual such as -
people with common interest living in a particular area.
group of people with a common characteristic or interest living together within a larger society.
A body of persons of common or professional interests scattered throughout a larger society.
A body of persons or nations having common history or common social, economic and political interests.
Agreements
- We are worthy of loving care
- we deserve to be whole
- we are capable of nurturing and supporting ourselves
- we can accept care from and offer care to others freely
Mind, body, spirit and community are the foundational elements of self-care.
To care for yourself sustainably, you must first know yourself.
Mind - what things help your brain feel happy and healthy? Sleep, Nutrition, exercise, meaningful work, medication
Body - what does your body need to function well? Sleep, Nutrition, exercise, regular cleaning, assistive devices, medication.
Spirit - what does your spirit need to feel peacefully energized? Hobbies, playtime, engaging work, quiet time, choose your own adventure.
Community - where, how and when do you belong to and care for others? Family relationships, 2AM emergency friends, locality, affiliations of education, religion, profession, familiar strangers.
Carbon
Interval
$record->published_at->diffForHumans();
$diff = $record->published_at->diffAsCarbonInterval();
$diff->totalDays // show total days
$intervalA = CarbonInterval::minutes(30)
$intervalB = CarbonInterval::minutes(10)
$intervalC = CarbonInterval::minutes(20)
$intervals = [$intervalA, $intervalB, $intervalC];
usort($intervals, [CarbonInterval::class, 'compareDateIntervals]);
Period
foreach ([2020,2021,2022] as $year) {
$weeksInYear = Carbon::create($year)->isoWeeksInYear
foreach(range(1,$weeksInYear) as $week) {
$startOfWeek = Carbon::now()->setISODate($year, $week)->startOfWeek();
$weeks[$startOfWeek->format('Y-W')] = $startOfWeek->format('Y-m-d (W)');
}
}
OR
$carbonPeriod = CarbonPeriod::since('2020-01-01')
->until('2022-21-31')
->weeks(1);
foreach ($carbonPeriod as $week) {
$week[$week->startOfweek()->format('Y-W')] = $week->format('Y-m-d (W)');
}
Ui Testing
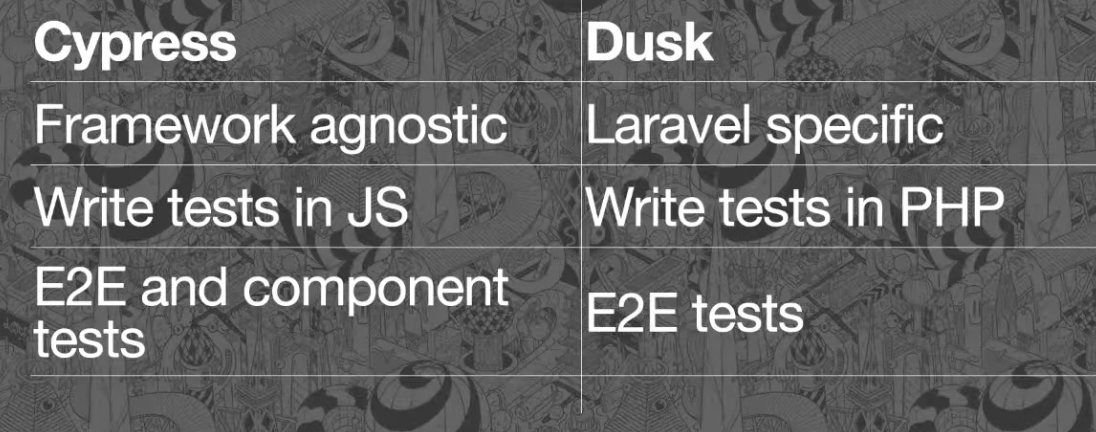
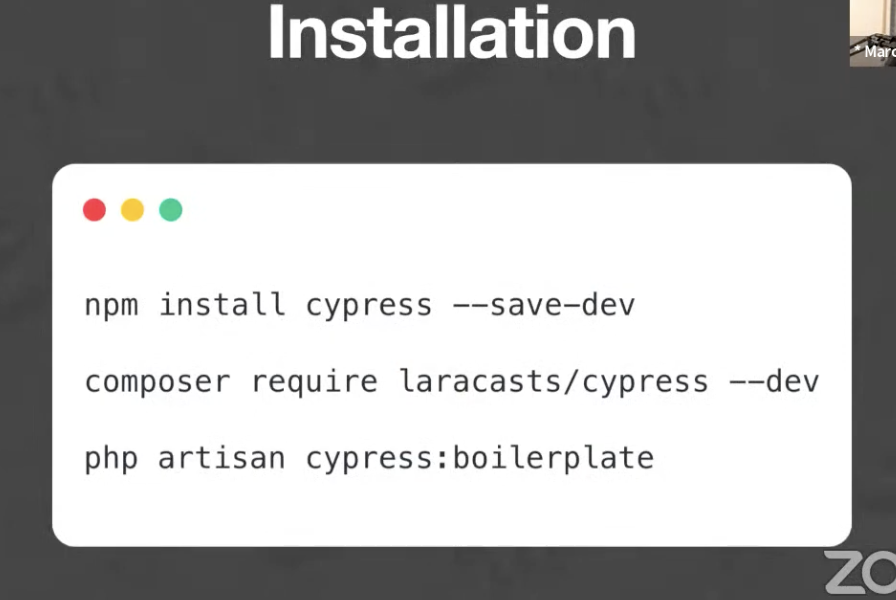
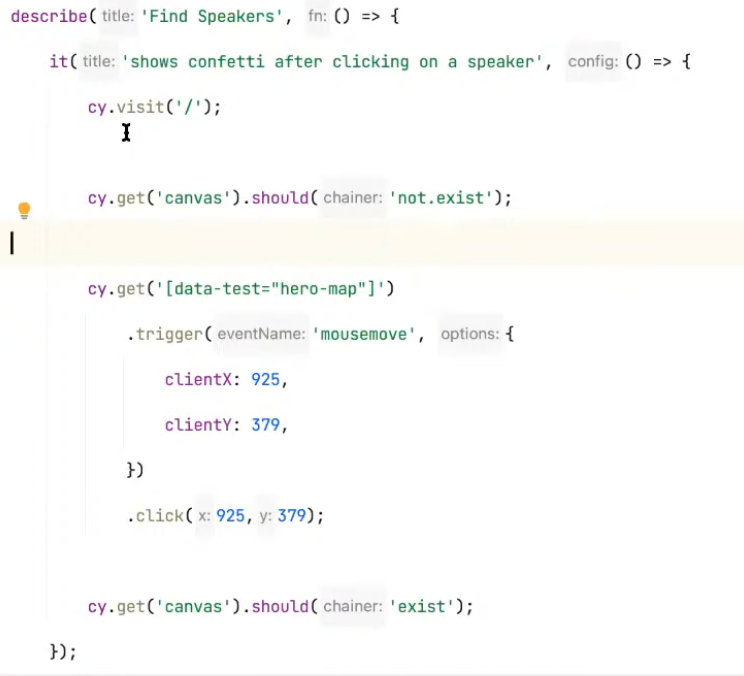
npx cypress open
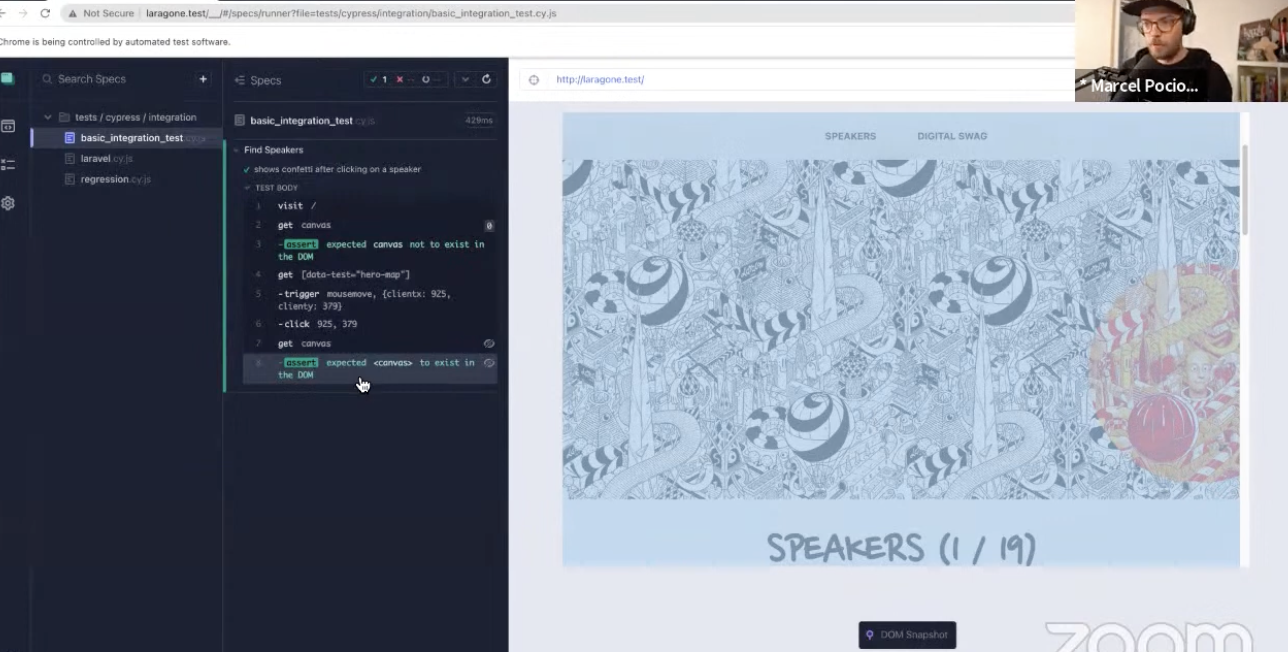
https://github.com/laracasts/cypress
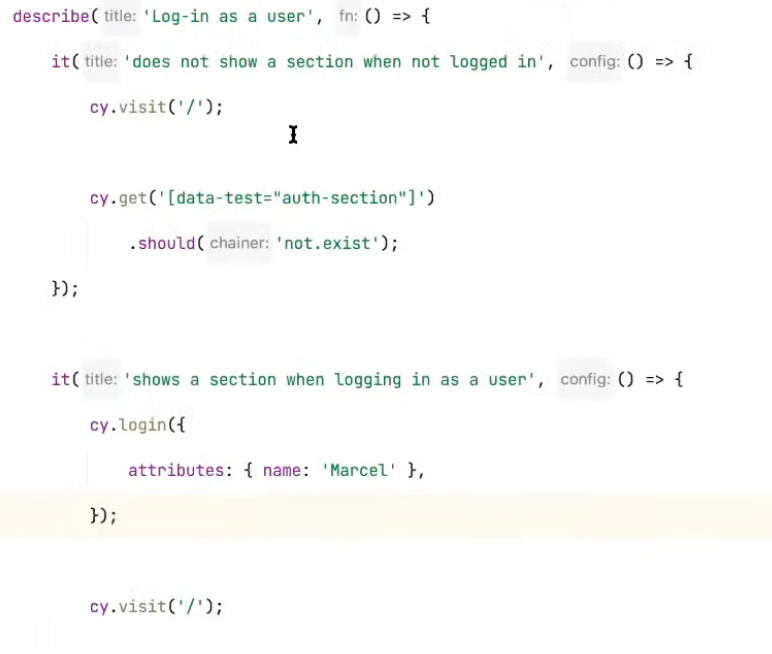
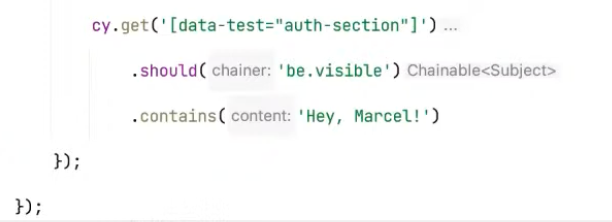
Abstracting / Complicating too early
Yagni : the basic
- YAGNI means “you aren’t gonna need it”
- we suck at predicting the future
- counter to: control against bad outcomes with abstractions and planning.
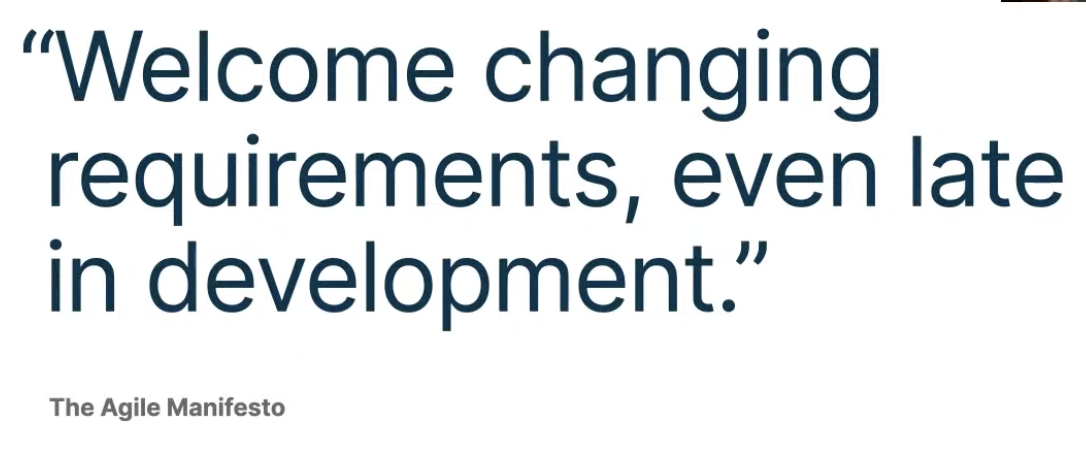
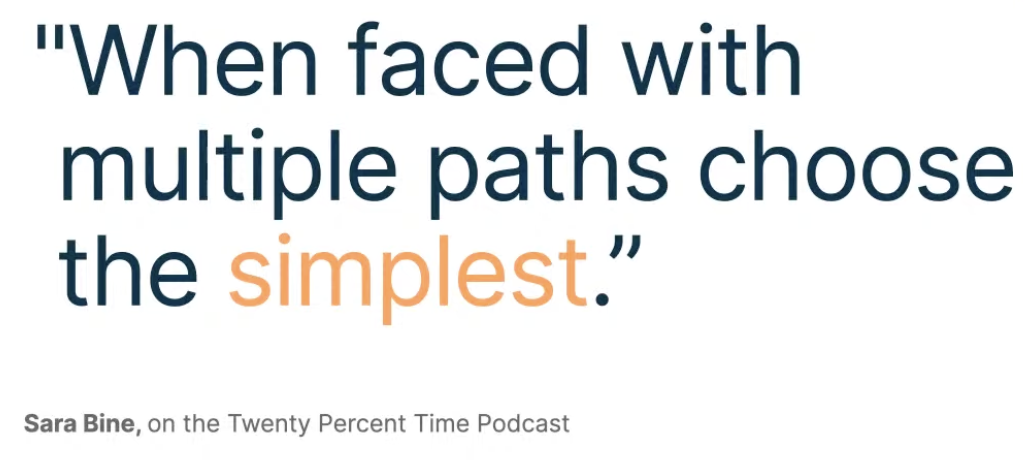
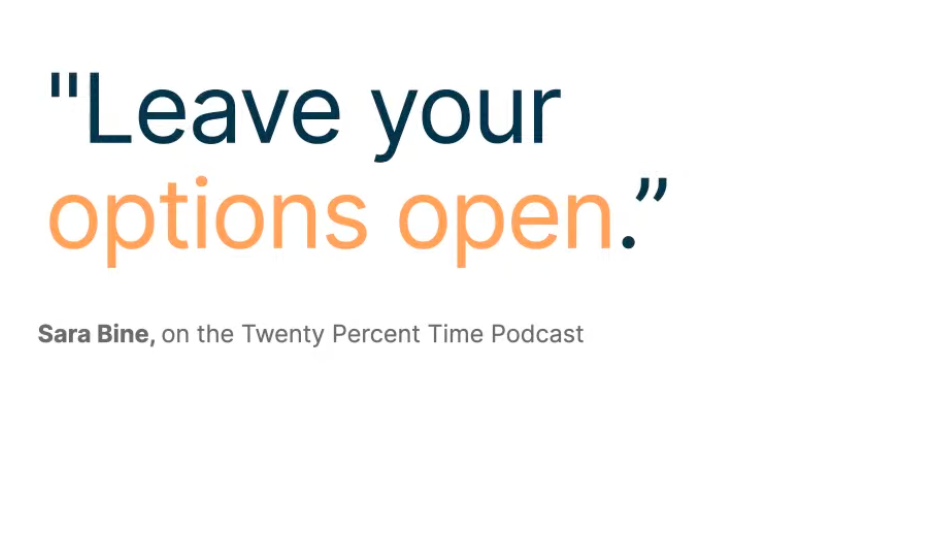
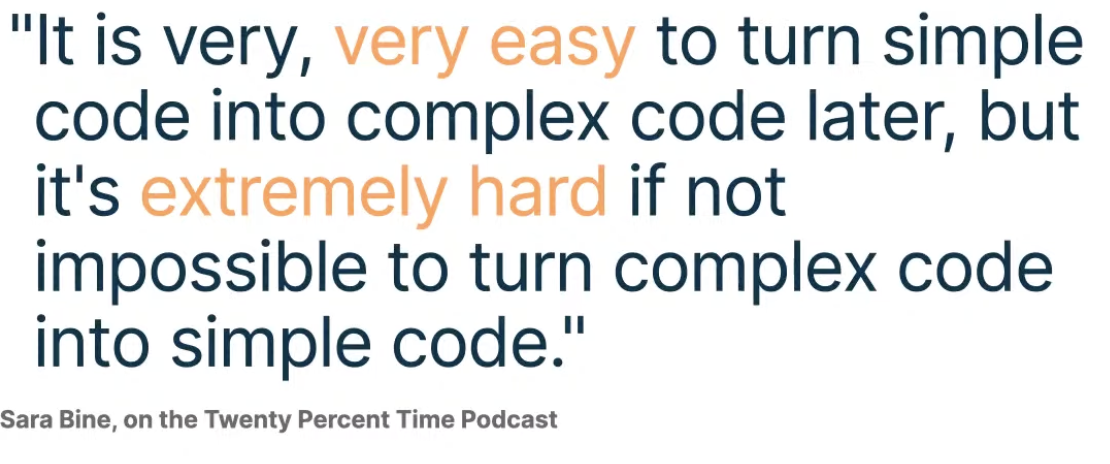
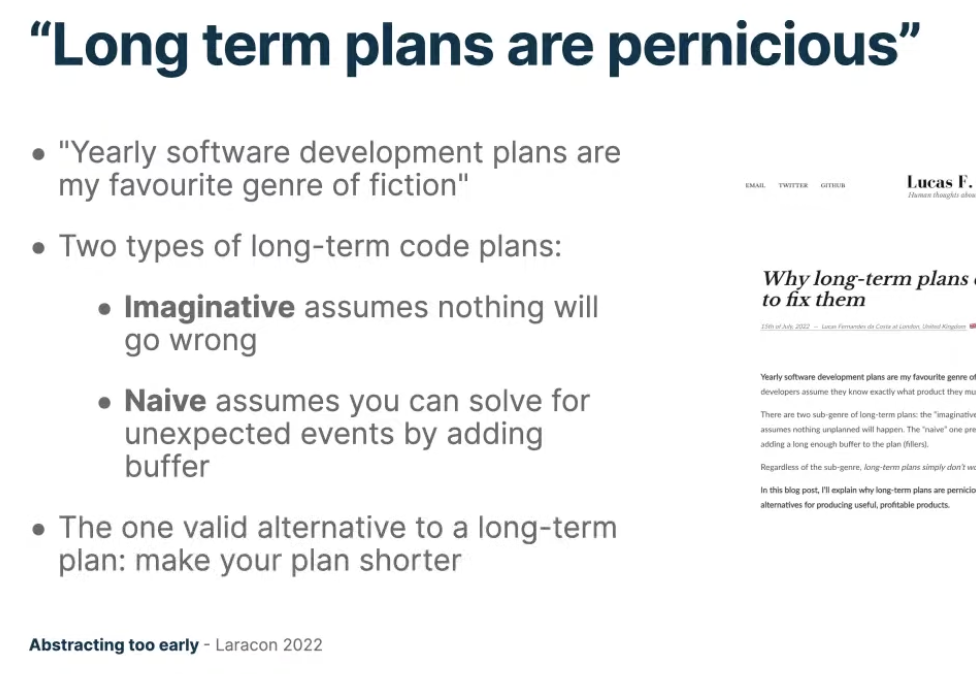
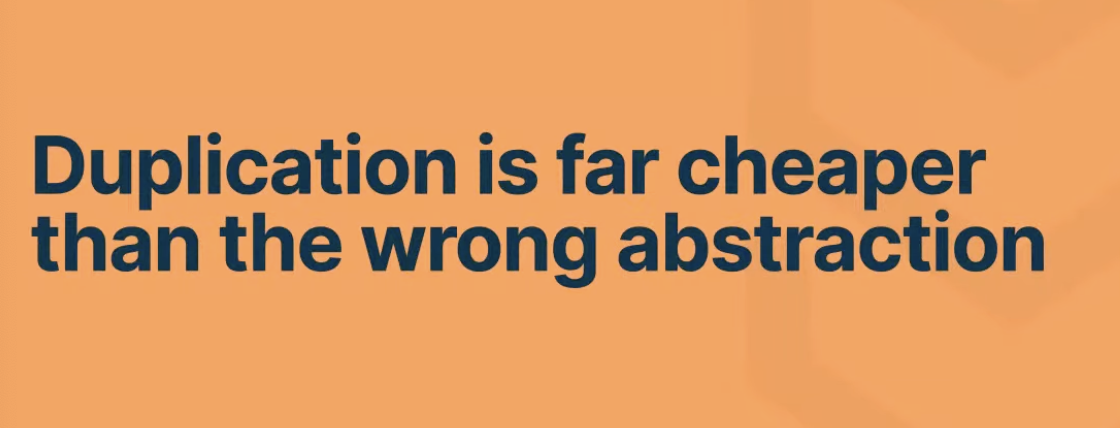
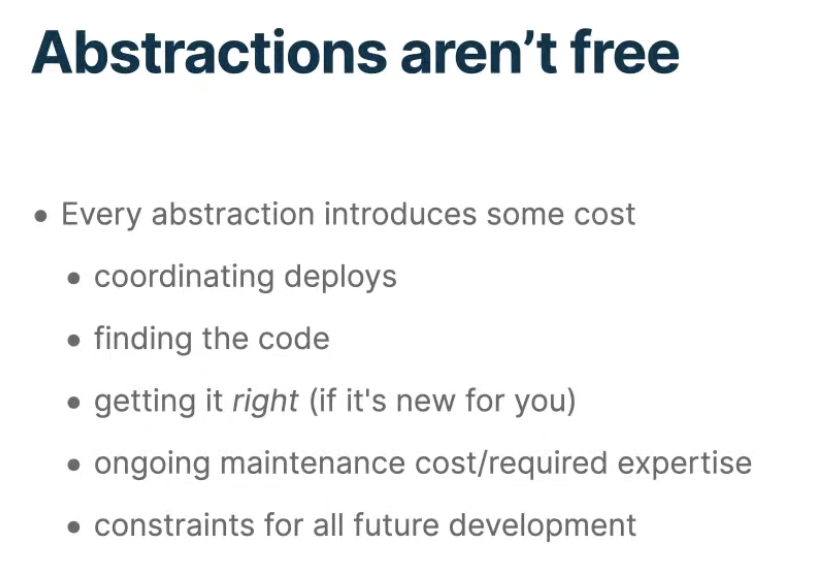
Aaron Francis
Schema
Small - as small as you can get away with as big as you need,
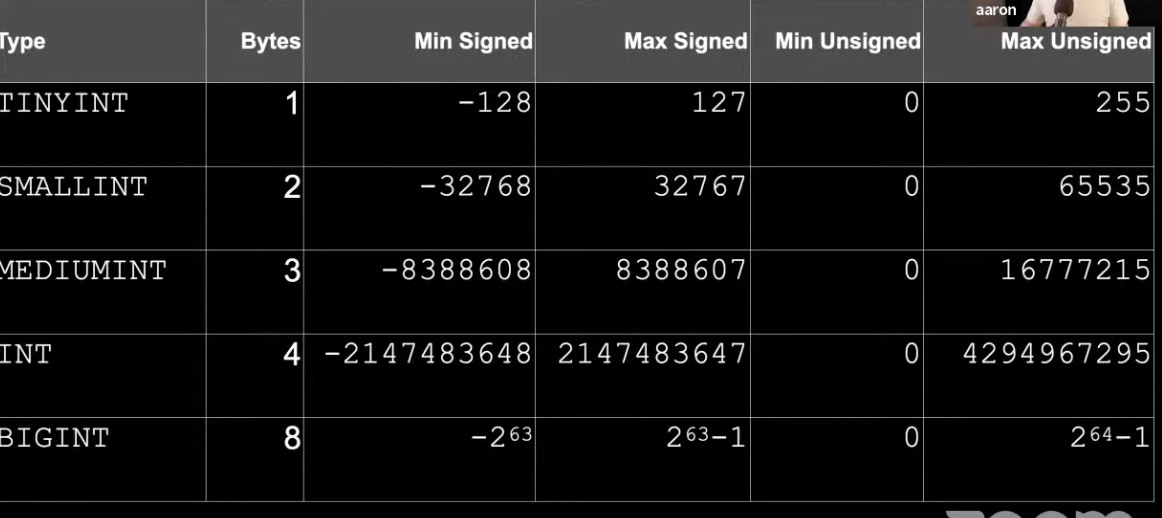
Simple - prefer integers to string, prefer dates to string blob < varchar < char,
Honest - avoid null, but don’t lie match reality
Indexes
B-tree indexes
- a separate data structure
- a copy of part of your data
- have a pointer to the row
Data → Schema
Queries → Indexes
Designing Indexes
- as many as you need
- as few as you can get away with
Rules for composite indexes
- Left to right no skipping
- Stops at a range
Covering indexes - regular index in a special situations.
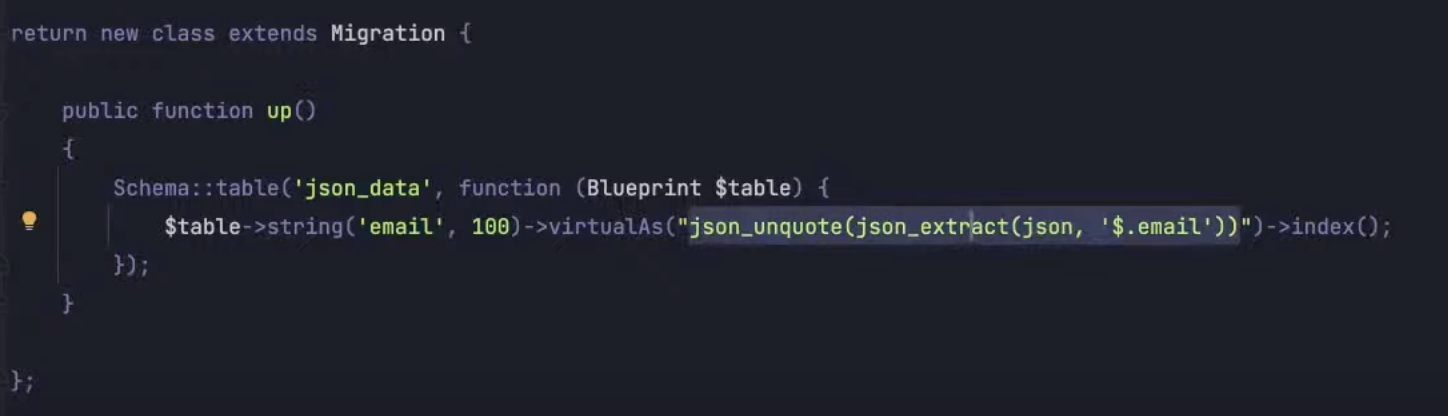
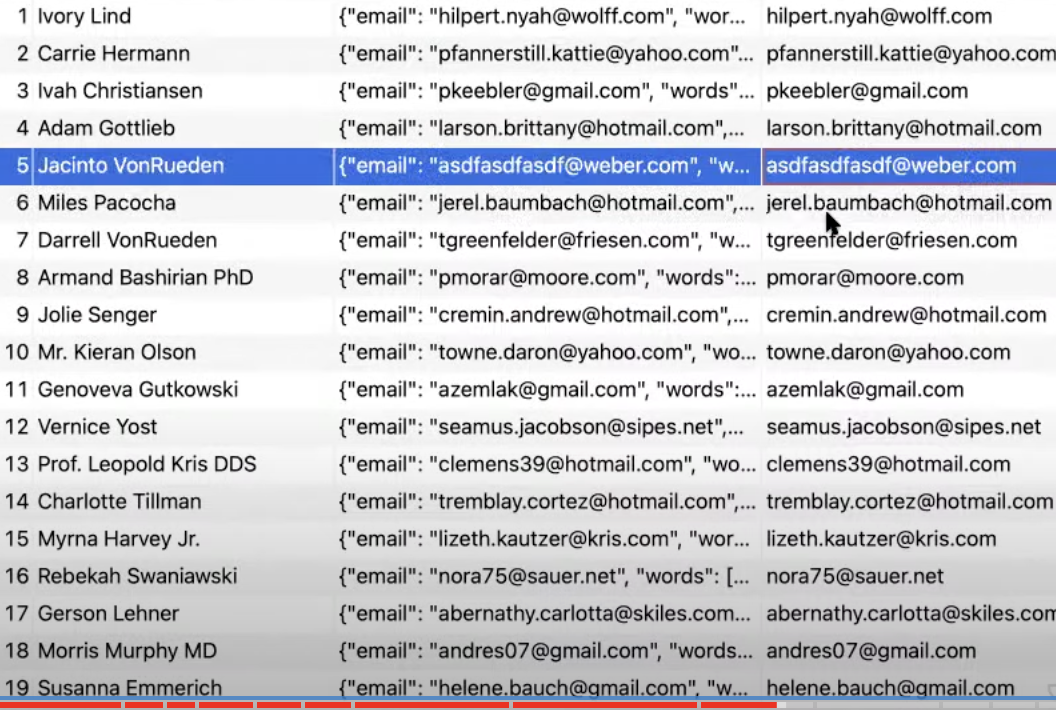
Queries
Don’t obfuscate your indexes
select only what you need
subquery =! bad
Git interactive
git rebase -i {commit}~
Meaningful Mentorship
What does it mean to be a mentor?
Mentorship is the opportunity to share expertise and help others break into the industry, learn a new skill, or otherwise advance their career.
Why become a mentor?
- To give back to the community
- To help your coworkers or new employees onboard new technologies or projects.
- “Because someone did it for me”
5 quick tips to become an effective mentor
- Build the relationship.
- The most important part of being a meaningful mentor.
- Mentorship requires trust in both directions.
- Start by scheduling a consistent time to meet on a recurring basis that works for both of you.
- Practice empathy
- Take a walk in your mentee’s shoes.
- Remember that you were a beginner once, too.
- Affirm frustrations and difficulties, and celebrate victories and successes.
- Level up with pair programming.
- Great way to build an understanding of technical topics or complex sections of code.
- Build mentee confidence
- “Gradual release of responsibility”
What is the gradual release of responsibility?
Education model
I do → we do → you do
Pair programming model
I talk I type → I talk, You type → You talk, I type → You talk you type
- Encourage them to set concrete goals
- Concrete goals have a defined end with tangible output.
- Advise your mentee to choose reasonable goals.
- Celebrate reaching the goals, no matter the size.
- Let your mentee lead
- Your mentee should drive the mentorship process
- Expect and embrace the fluidity
- Prefer advice and experience instead of answer and solution.