Takehome Anime Project & Interview
The assignment is simple but challenging enough to make, it consists of making a list of anime with pagination, searching for an anime, and having the detail page. The desired goals were Quality of code, scalable and maintainability, have a good experience in searching and viewing data. Requirements are convenient UX, unit tests, using nextJS or reactJS, historical and atomic commits, instructions for use at READ.md, and explanation at EXPLANATION.md. The bonuses are caching, offline capability, BEM standardization, responsiveness, and containerized apps.
API Resource: https://docs.api.jikan.moe/#tag/anime/operation/getAnimeSearch
First I checked which task needed to be taken down, for me it was listing the data, showing the data, and searching the data. Refactor all the code that can be moved to components while fixing the UI, then add some unit tests (didn't know what to use, so just checked the documentation, it's either Jest or Vitest). Jest was too complicated to start with so I used Vitest which I think is a much simpler way to make unit tests.
Atomic Commits
When possible, a commit should encompass a single feature, change, or fix. In other words, try to keep each commit focused on a single thing. This makes it much easier to undo or rollback changes later on. It also makes your code or project easier to review.
Result
Interview
This is what was asked for the interview, some of the questions couldn't be answered but I think I did pretty well.
Why is there a lifecycle in React app?
My answers were different, I chose to make an example of an Android app which have a lifecycle as well.
What are the differences between cookie, local storage, and session storage
Below are some of the differences between a cookie, local storage, and session storage,
Feature | Cookie | Local storage | Session storage |
---|---|---|---|
Accessed on client or server side | Both server-side & client-side | client-side only | client-side only |
Lifetime | As configured using Expires option | until deleted | until tab is closed |
SSL support | Supported | Not supported | Not supported |
Maximum data size | 4KB | 5 MB | 5MB |
After researching this is the difference between those 3, the cookie can be accessed by the server and client, the rest client side only, for lifetime cookie is configured using Expires option, local storage until deleted, session until tab is closed.
What is Hoisting
Hoisting is a JavaScript mechanism where variables, function declarations, and classes are moved to the top of their scope before code execution. Remember that JavaScript only hoists declarations, not initialization.
console.log(message); //output : undefined
var message = "The variable Has been hoisted";
Let's take a simple example of variable hoisting,
var message;
console.log(message);
message = "The variable Has been hoisted";
In the same fashion, function declarations are hoisted too
message("Good morning"); //Good morning
function message(name) {
console.log(name);
}
This hoisting makes functions to be safely used in code before they are declared.
What is the purpose of the let keyword
The let
statement declares a block scope local variable. Hence the variables defined with let keyword are limited in scope to the block, statement, or expression on which it is used. Whereas variables declared with the var
keyword are used to define a variable globally, or locally to an entire function regardless of block scope.
Let's take an example to demonstrate the usage,
let counter = 30;
if (counter === 30) {
let counter = 31;
console.log(counter); // 31
}
console.log(counter); // 30 (because the variable in if block won't exist here)
What if there's a lot of data, how would you fetch and render that?
I explained about chunking data where the data after fetching it would show the first few data and then keep going, but after researching this maybe what he meant was probably parallel data fetching but this happens when there are different endpoints, I may be wrong.
What is the difference between grid and flexbox?
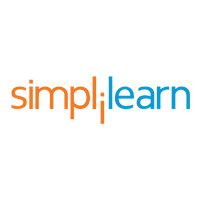
If there are non-compatible properties cross-browser how do you handle this?
What is virtual DOM?
Conclusion
This was my first frontend Interview and from the interviews I've taken, I can make a point that we will be better at taking interviews.