Design Pattern & SOLID Design
Finally, I get to learn about this pattern that haunts me, every time they talk about this and I had no idea what were they.
Design Pattern
- Decorator pattern
In my own word . this is like implementing an interface but it adds another subclass with a constructor if needed. when would be appropriate to use this pattern or maybe like inheritance? ask yourself if would you be needing the whole function of the class(inheritance) or maybe just to adjust a specific function.
- Adapter pattern
This is to make the same class but wrapped in different behavior. Like, open a book in real is is to open a book but to open a book in Kindle open book in Kindle way- the template method pattern. Extract the same method to an abstract class Abstract in a abstract class, Any subclass is required to get this method
- pick a strategy
define a family of algorithm2. encapsulate and make them interchangeable
- the chain of responsibility
gives us the ability of chain object calls while giving each object the ability to either end the execution and handle the request or if it can't handle the request it'll send of the request of the chain. Benefit to this approach when its relevant, the client can make a request without knowing how that request was made
- the specification pattern
It allows you to take any business rule and promote it to a first class citizen.
- observer pattern
the change in one object need to have a nice flexible decoupled way to notify other object of this change and that way they can respond how the need to.
Solid Design
- Single Responsibility
a class should have one, and only one, a reason to change
an object should only have a single reason to change
When there are multiple objects, you should extract them to a dedicated object
- Open closed
Entities should be open for extension but closed for modification
Change the behavior of the class without modifying source code
Avoid code rot
Separate extensible behavior behind an interface and flip the dependencies
whenever you find yourself type checking, you are breaking this rule
The goal is to get a point where you can never break core system
- Liskov substitution
Let q(x) be a property provable about object x of type T then q(y) should be provable for objects y of type S
where is a subtype of T
derived classes must be substitutable for their base class
1. signature must match
2. precondition can't be greater
3. post condition at least equal to
4. exception type must match simple explanation : the output should be same, or add blockdoc for output
- Interface segregation
a client should not be forced to implement an interface that it doesn't use
- Dependency inversion
Depend on abstraction, not on concretion
dependency inversion is not equal to dependency injection
all of this is about decoupling code
high-level code isn't as concerned with detail
slow level code is more concerned with details and specifics
Singleton
it's a type of object that can only be instantiated once. If the instance has already been created and if not it will create a new one and that ensures that only one object can be created. try to lean on your language's built-in features before implementing a fancy design pattern
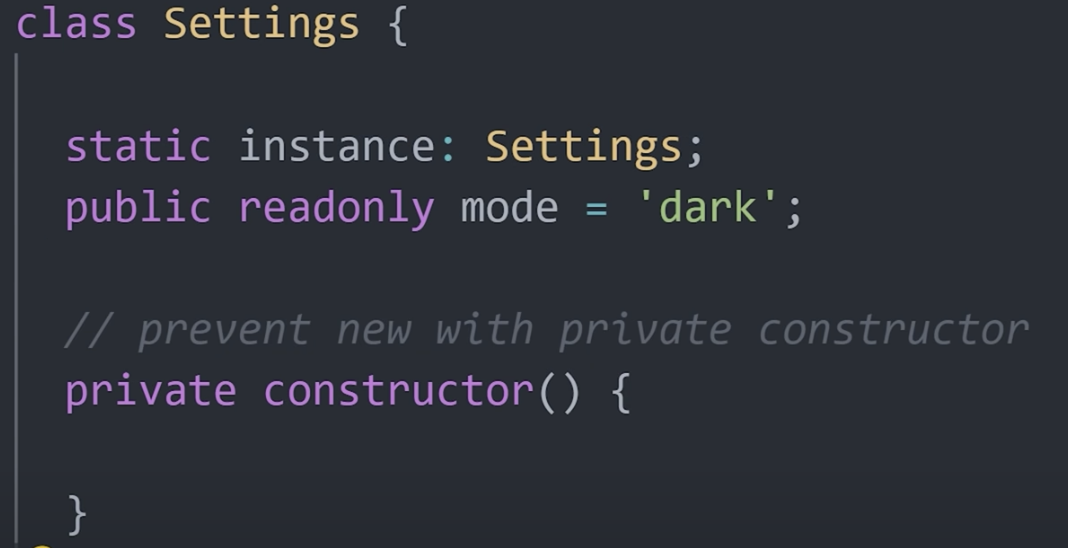
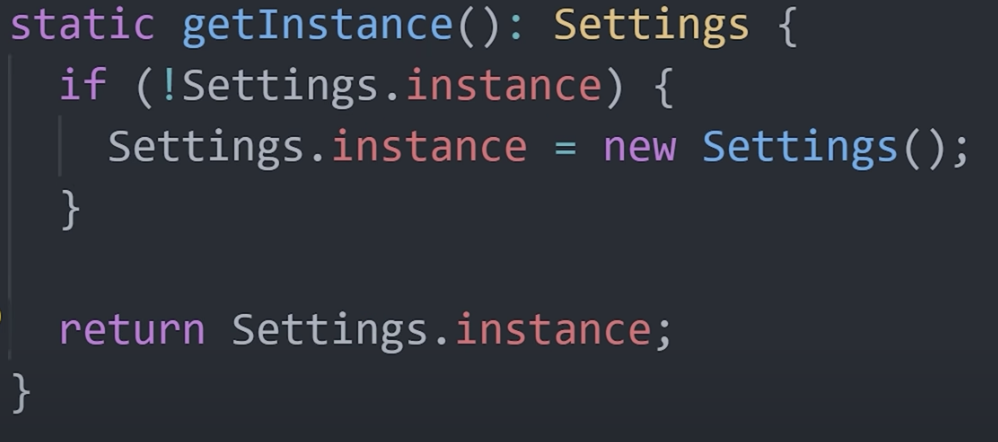
Prototype
AN Example of OOP, that can lead to a complex hierarchy
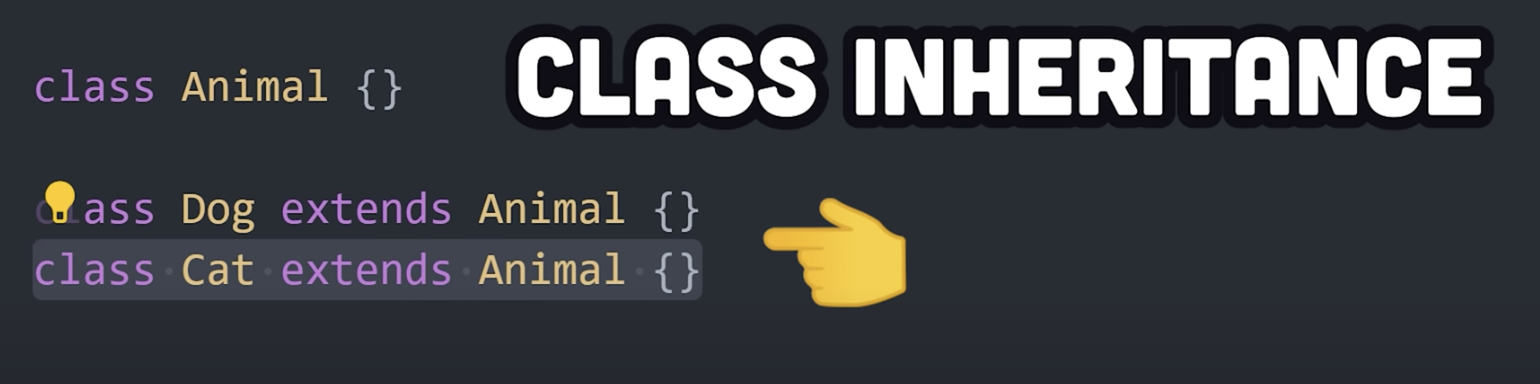
The prototype pattern is an alternative way to implement inheritance but instead of inheriting functionality from a class, it comes from an object that's already been created. This creates a flat prototype chain that makes it much easier to share functionality between objects, especially in a dynamic language like javascript which supports prototypal inheritance out of the box.
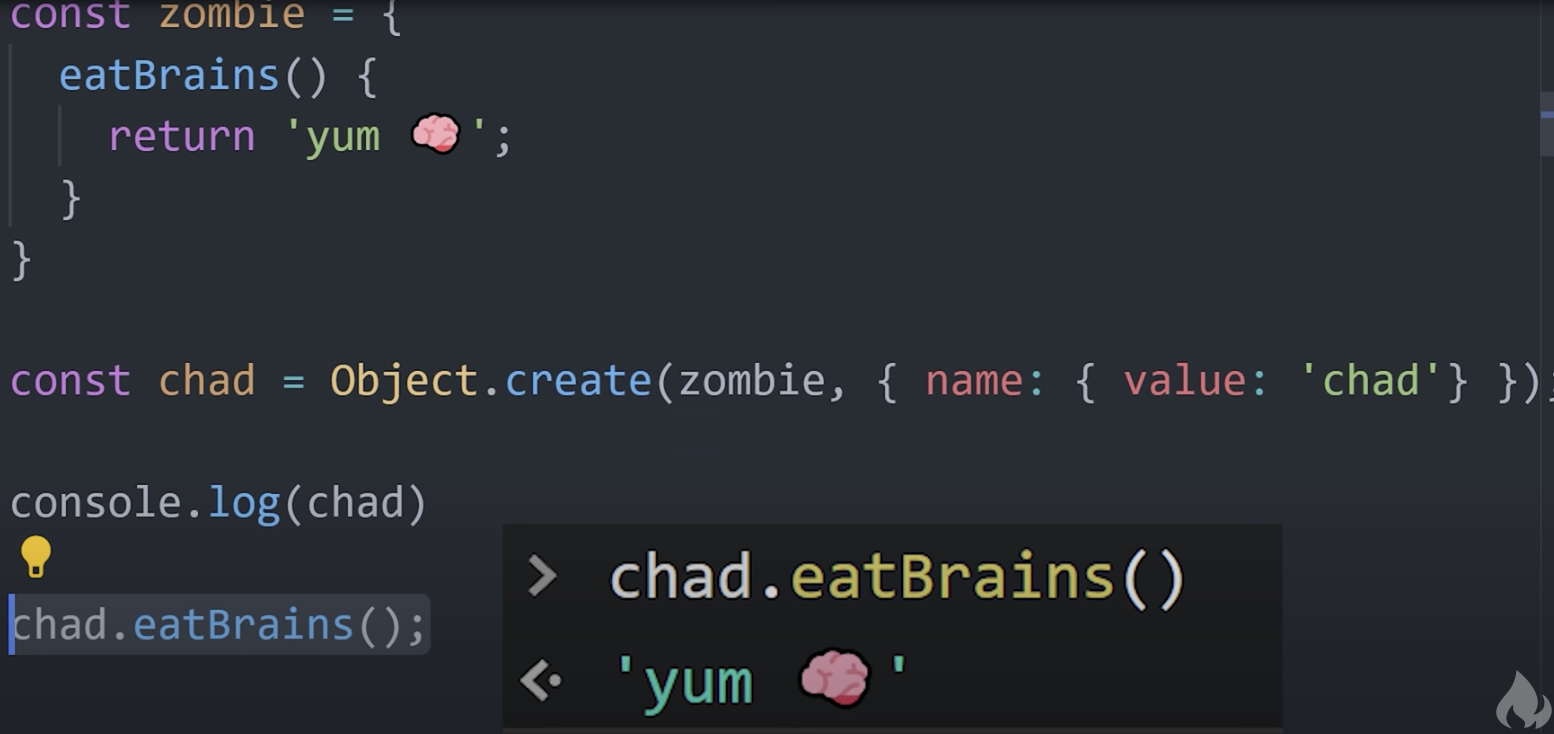
Builder
it's kind of hard to keep track of all these options and we might want to defer each step to a later point
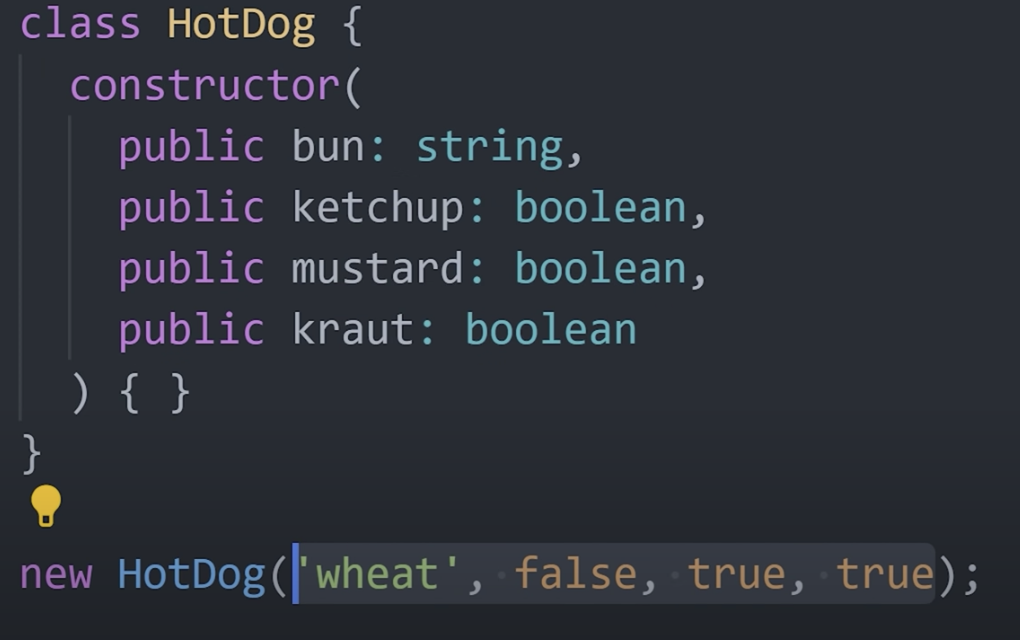
with the builder pattern, we create the object step by step using methods rather than the constructor and we could even delegate the building logic to an entirely different class in javascript we'll have each method return this which is a reference to the object instance that allows us to implement method chaining where we instantiate an object then chain methods to it but always get the object as the return value
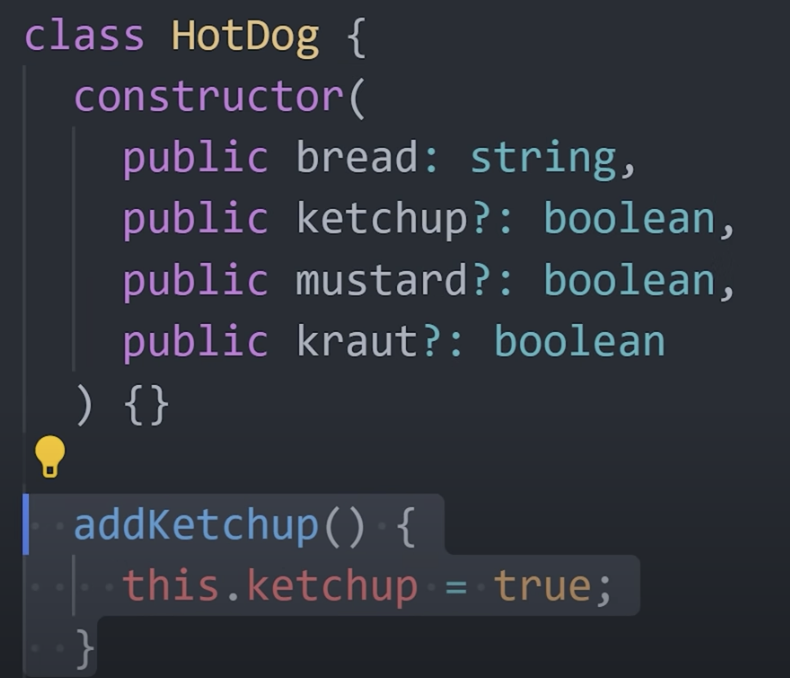
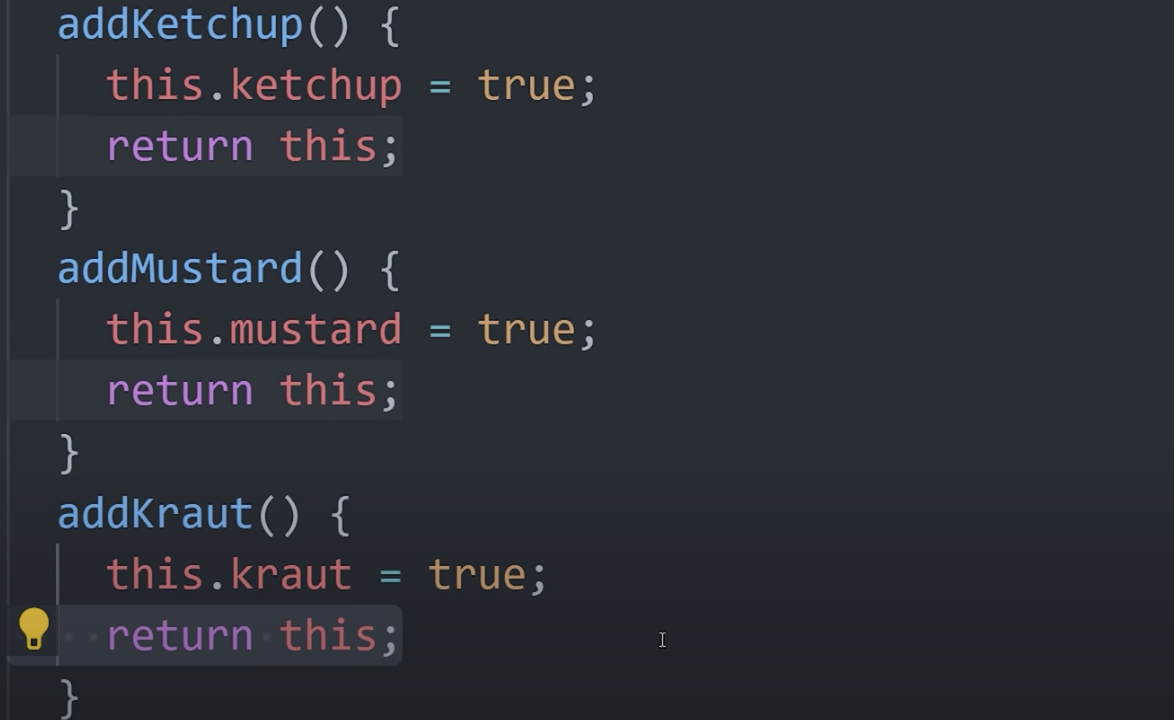
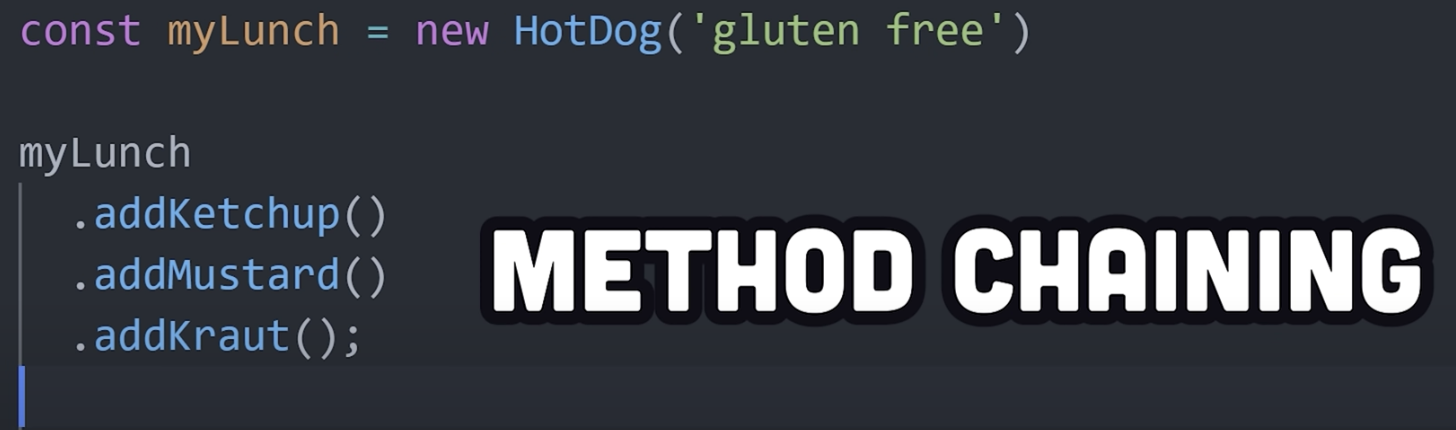
Factory
Instead of using the new keyword to instantiate an object you use a function or method to do it for you.
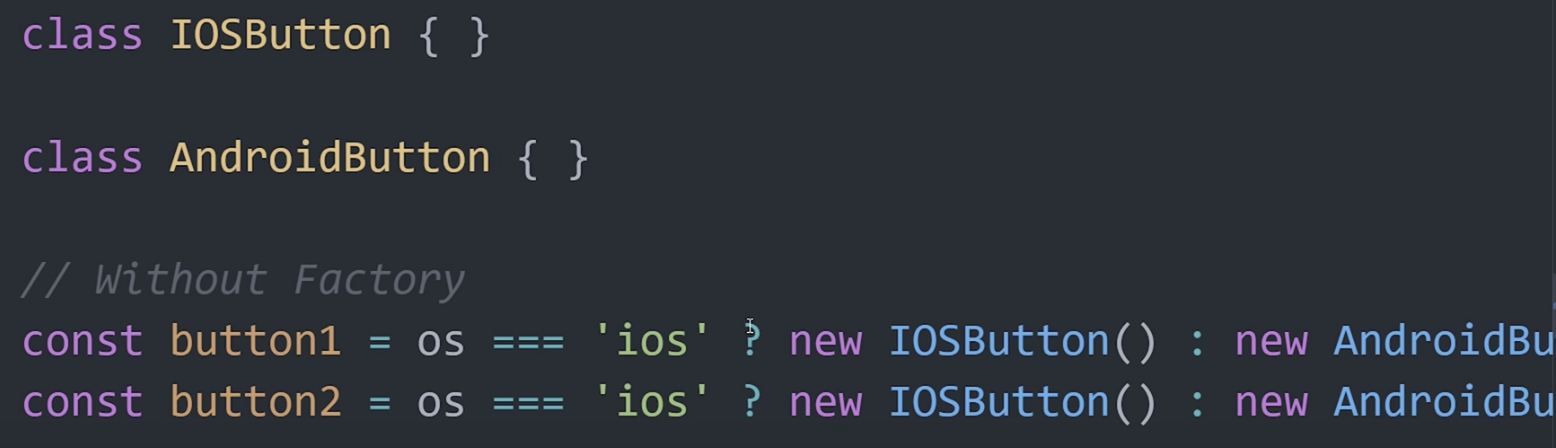
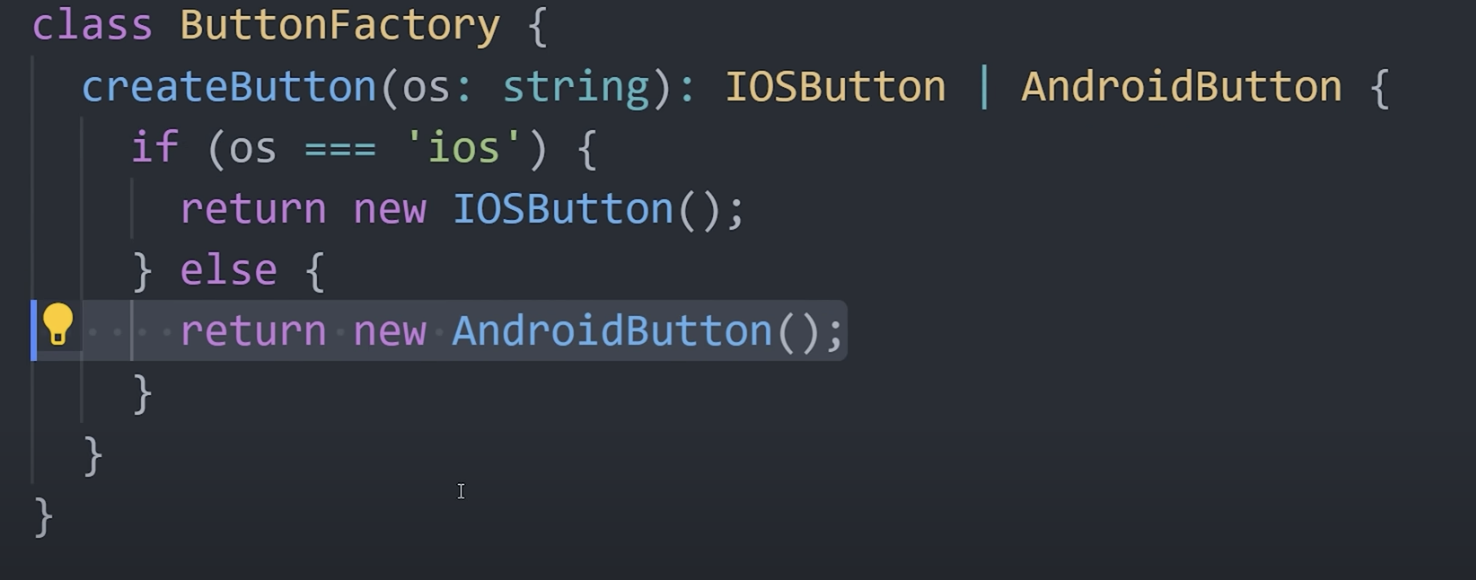
Fascade
A facade is the face of a building inside that building there are all kinds of shenanigans corruption and complexity that the end user doesn't need to know about.
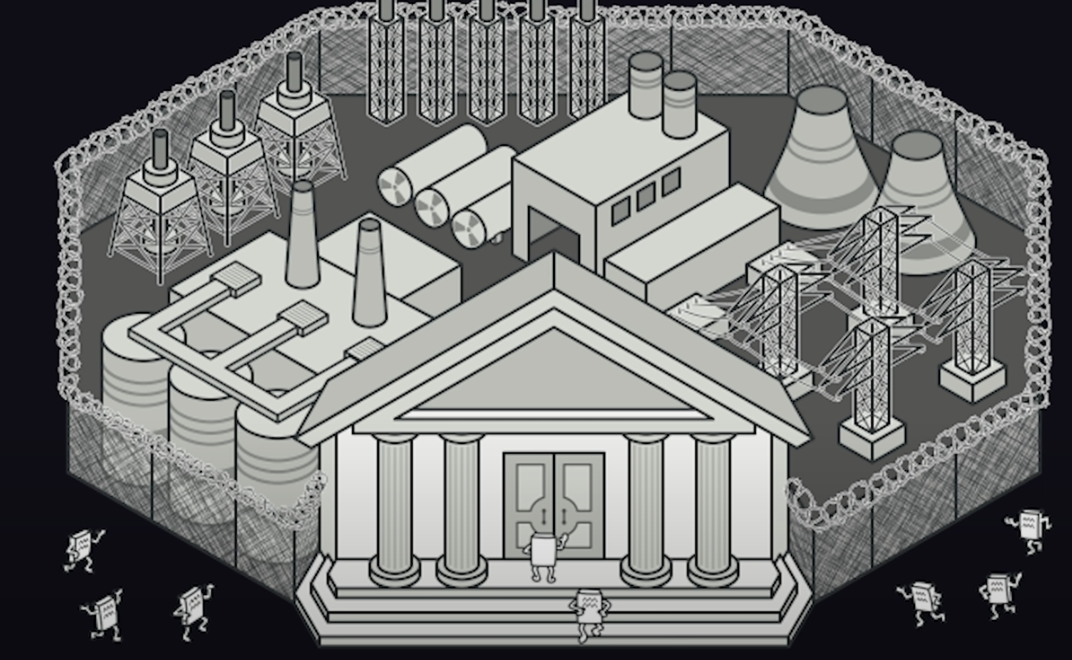
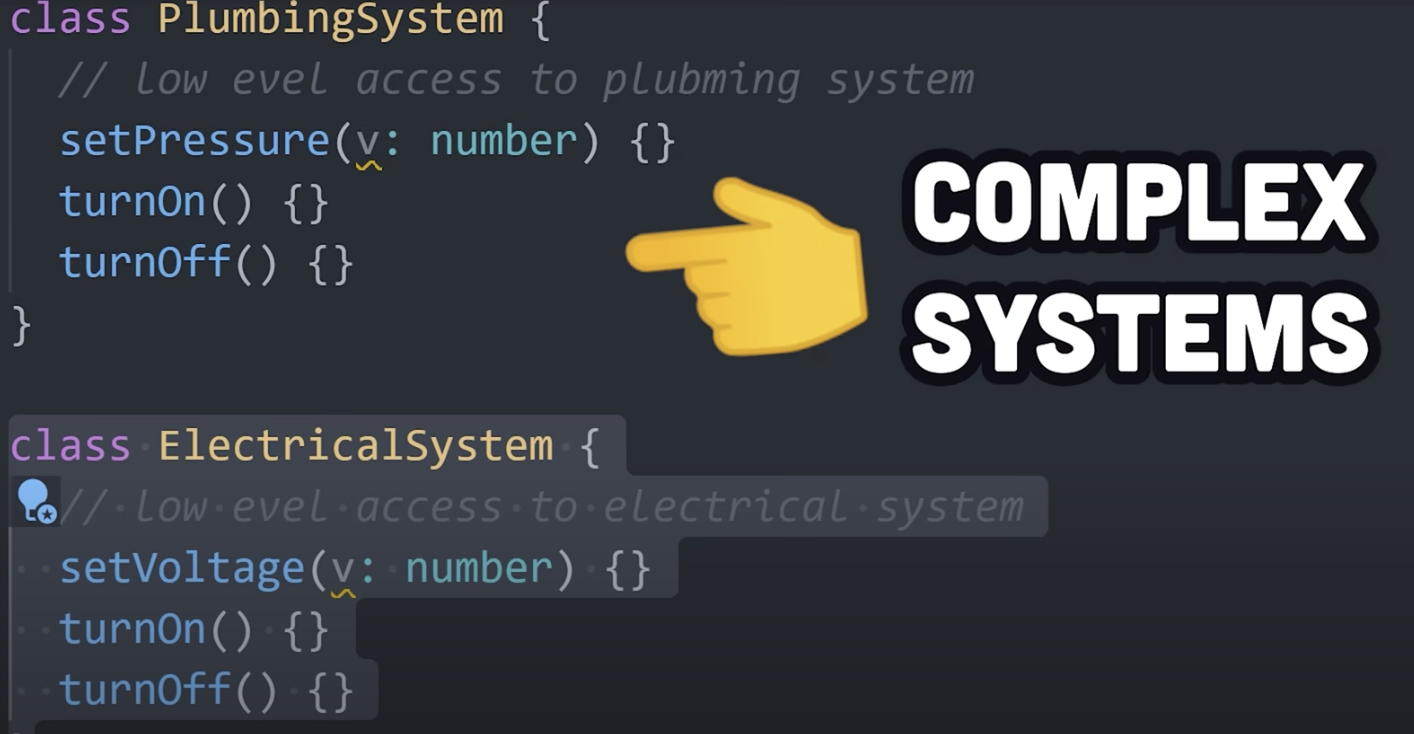
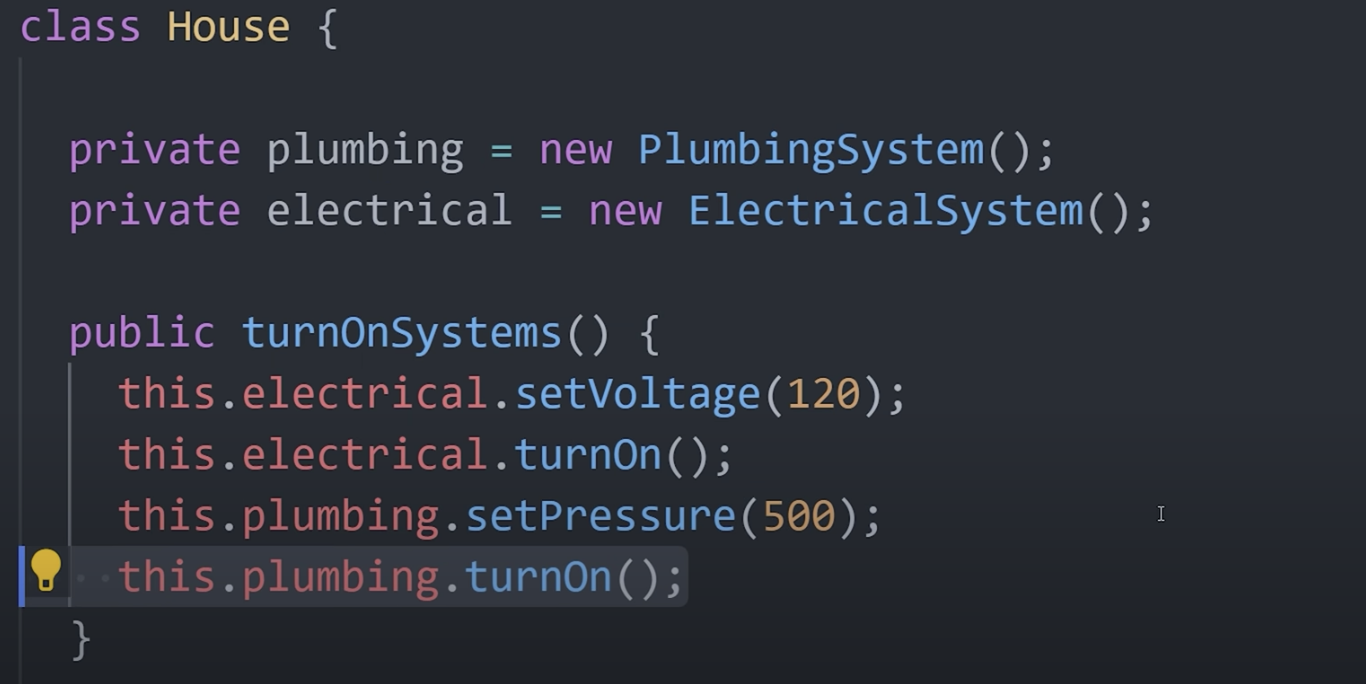

Proxy
Proxy is just a fancy word for a substitute like in school you might have a substitute teacher to replace the real thing in programming you can replace a target object with a proxy
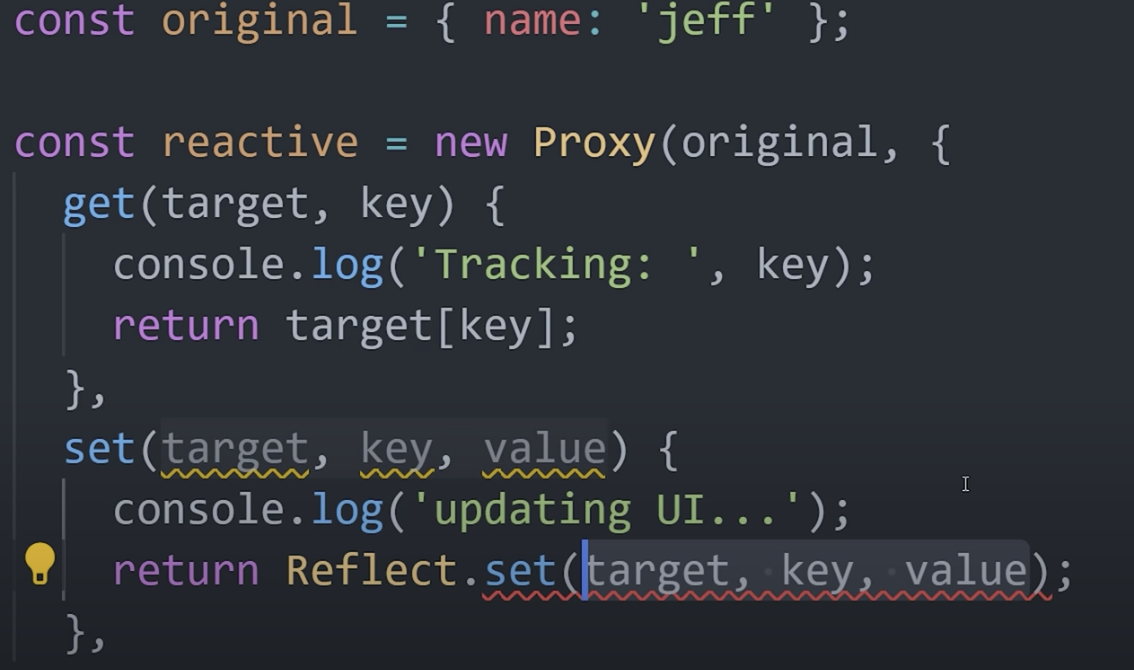
Iterator Behavioral
the iterator pattern allows you to traverse through a collection of objects modern languages already provide abstractions for the iterator pattern like the for loop when you loop over an array of items you're using the iterator pattern.
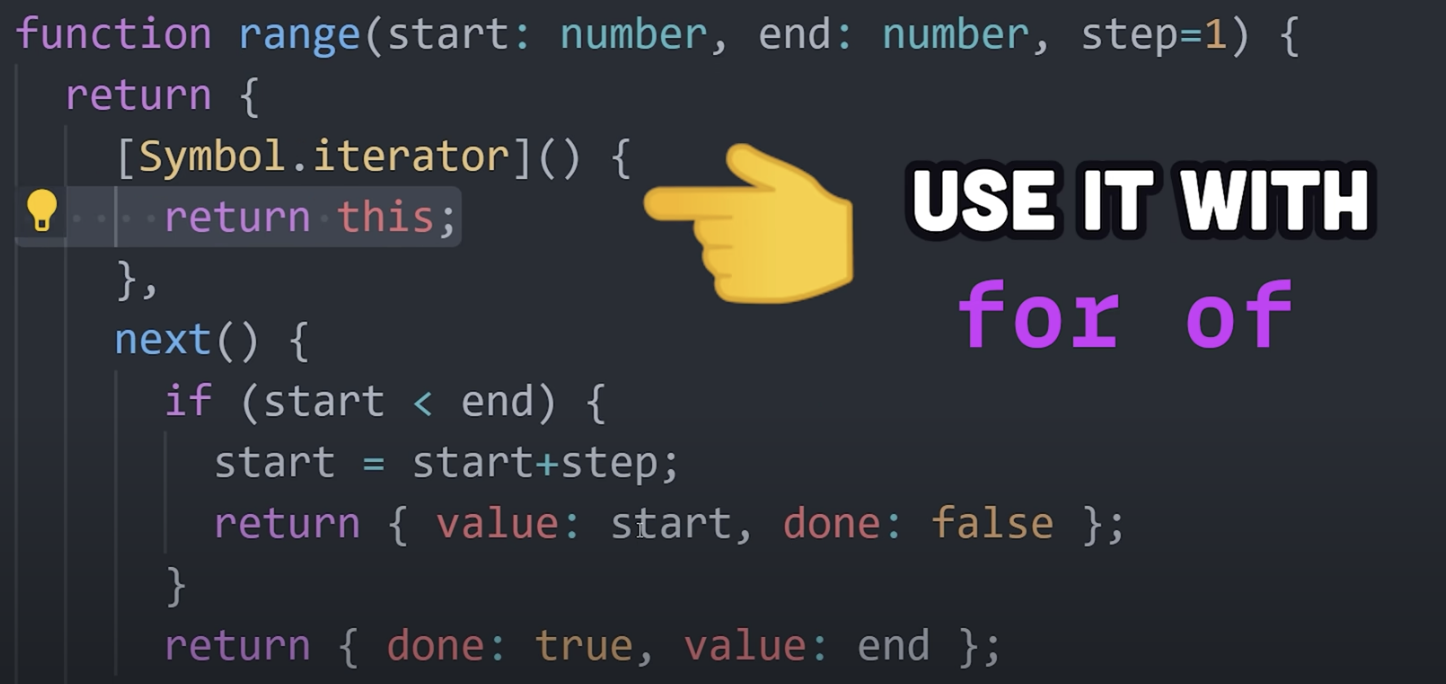
Observer Behavioral
observer which is a push-based system the observer pattern allows many objects to subscribe to events that are broadcast by another object it's a one-to-many relationship.
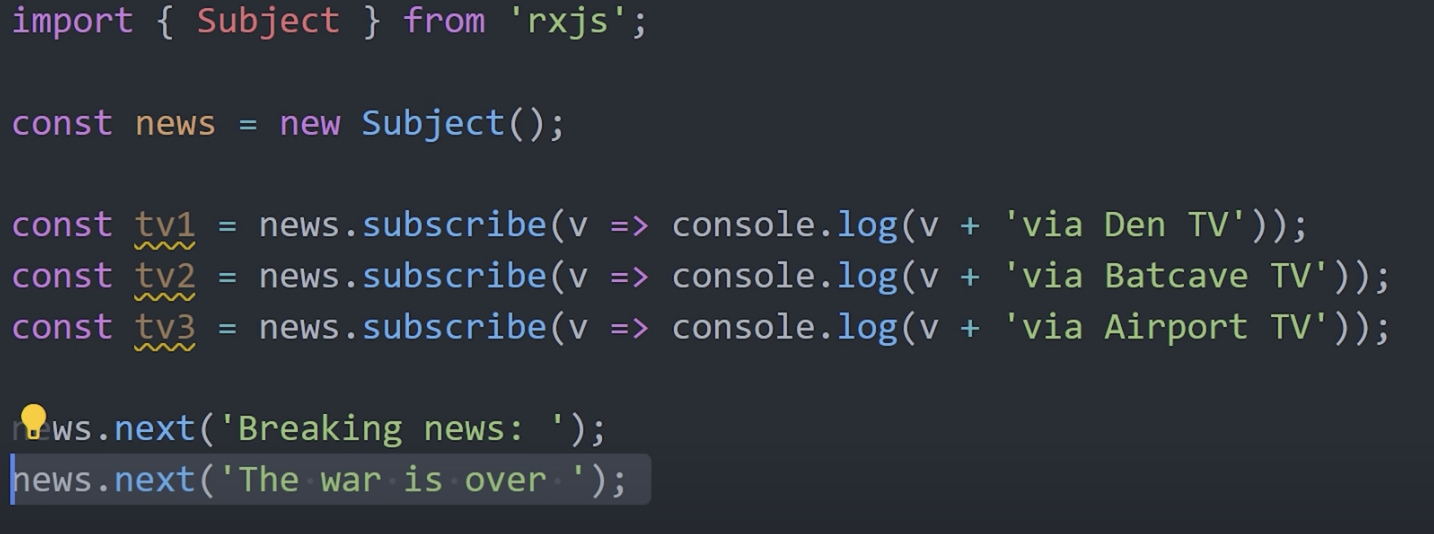
Mediator Behavioral
a mediator is like a middleman or a broker
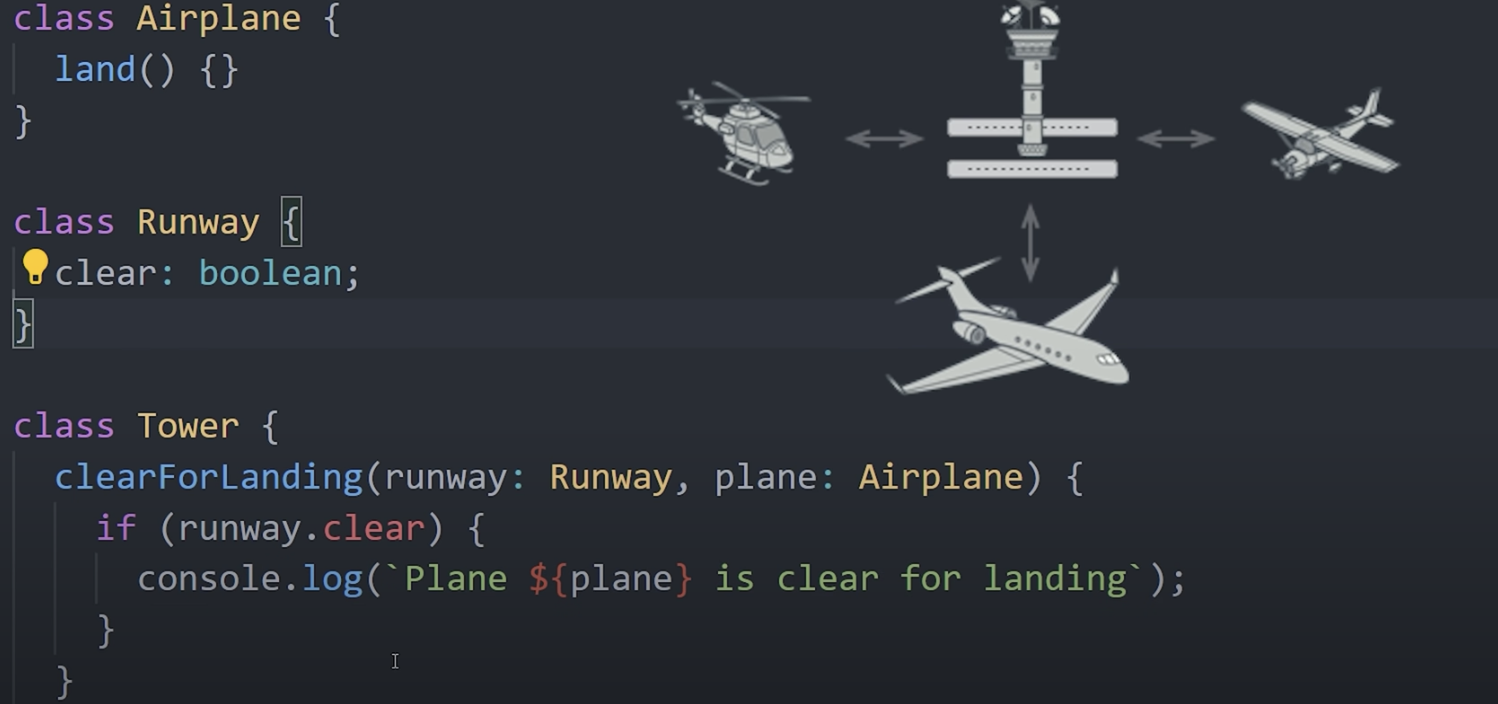
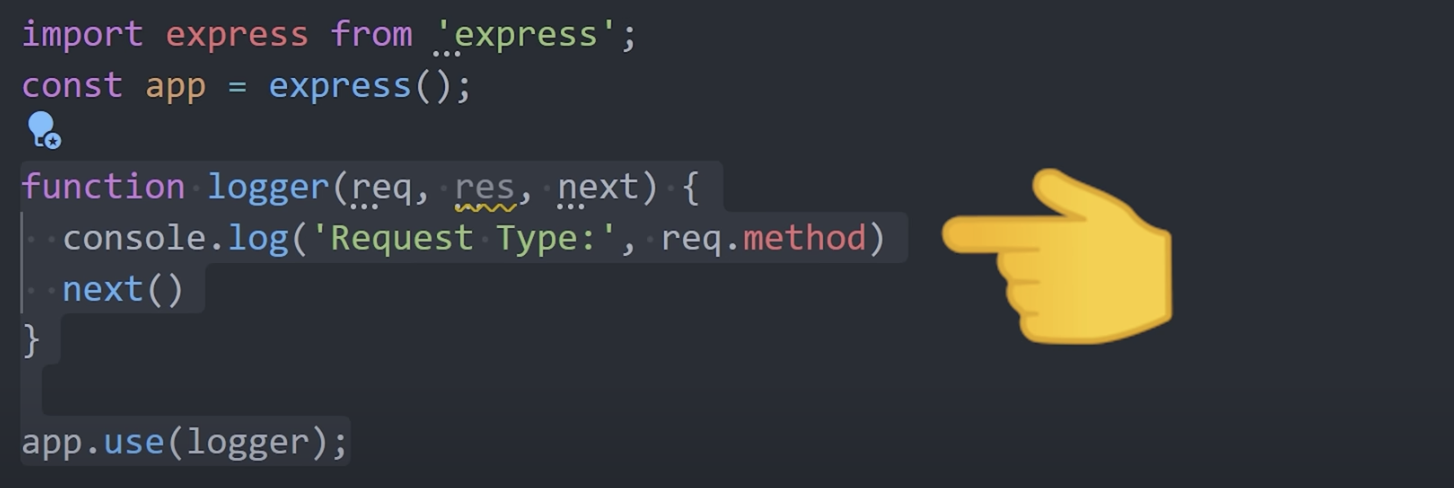
State Behavioral
Design pattern state where an object behaves differently based on a finite number of states in your code
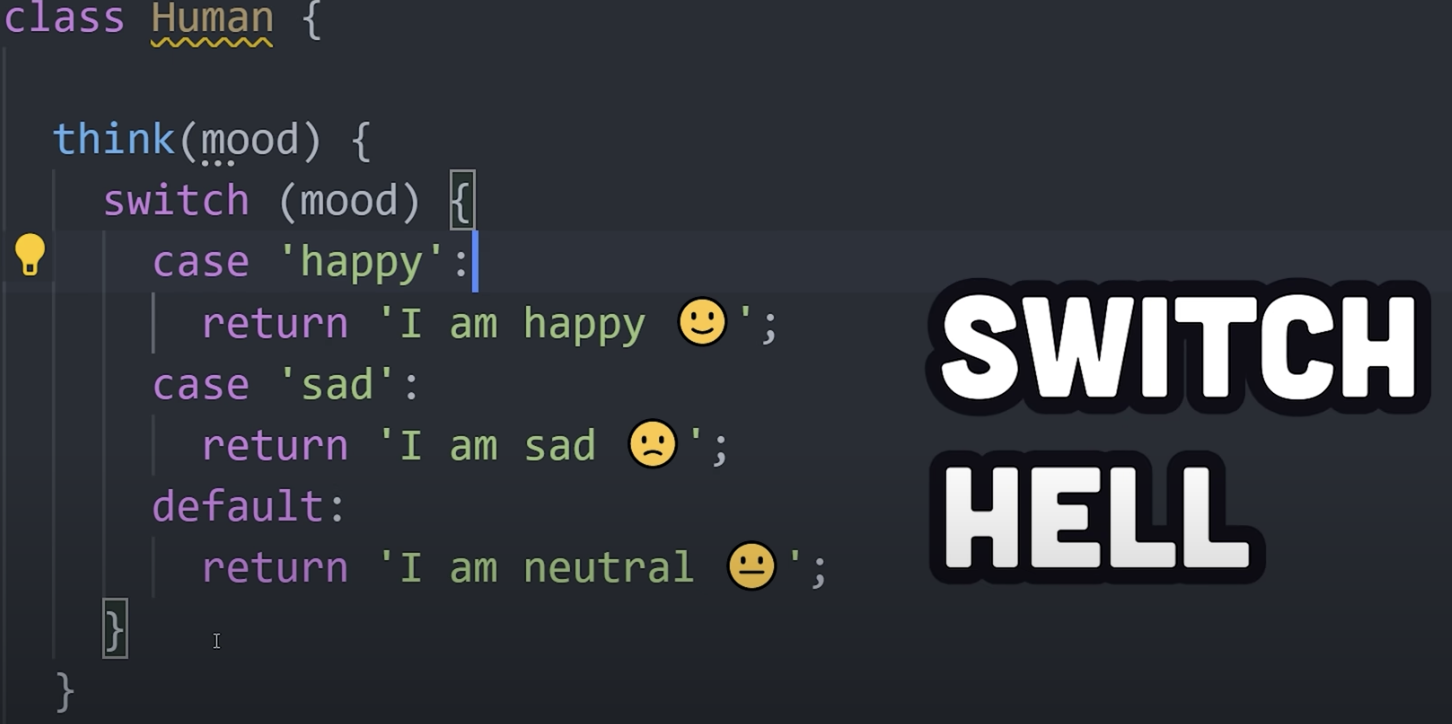
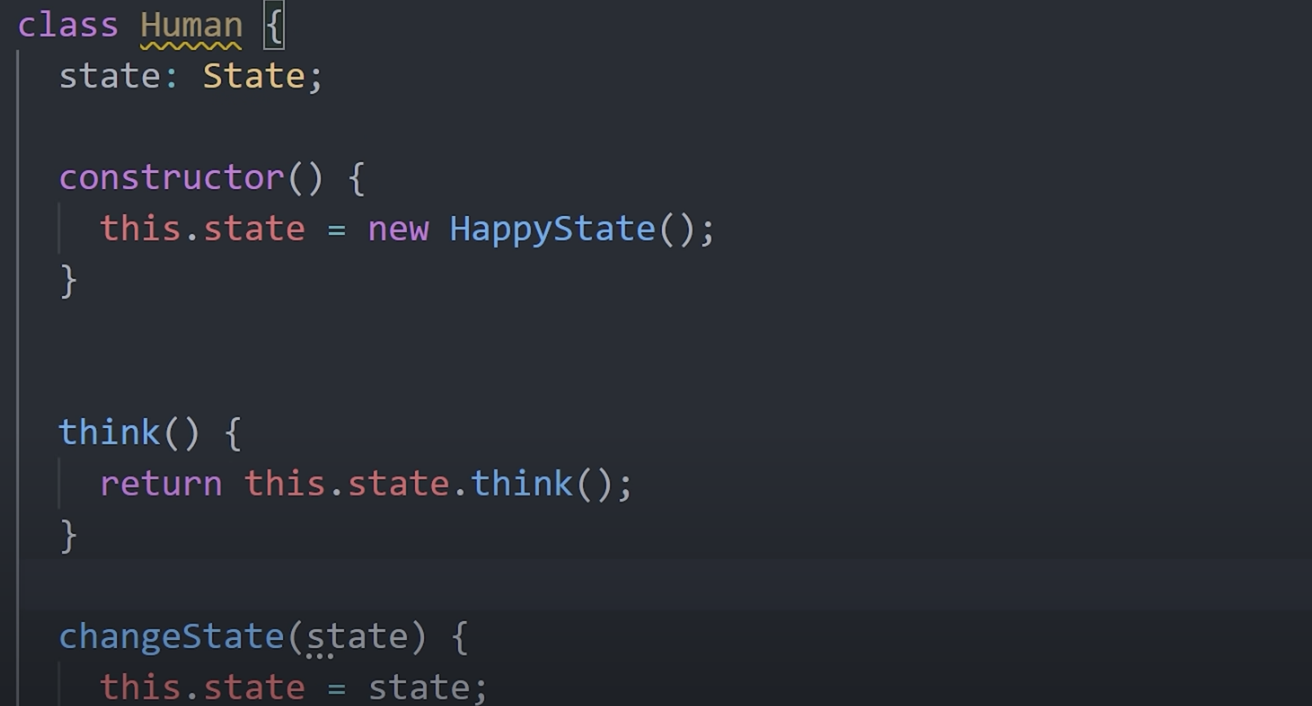